**myExceptions.h**
#pragma once
#include<iostream>
using namespace std;
#include<string>
class illegalParameterValue {
public:
illegalParameterValue(string theMessage = " Illegal Parameter Value") {
message = theMessage;
}
void outputMessage() { cout << message << endl; }
private:
string message;
};
class illegalIndex {
public:
illegalIndex(string theMessage = " Illegal Index") {
message = theMessage;
}
void outputMessage() { cout << message << endl; }
private:
string message;
};
**linearList.hpp**
#pragma once
#include<iostream>
using namespace std;
template<class T>
class linearList {//抽象类
public:
virtual ~linearList() {}//虚析构函数
virtual bool empty() const = 0;
//返回ture,当且仅当线性表为空
virtual int size() const = 0;
//返回线性表的元素个数
virtual T& get(int theIndex) const = 0;
//返回索引为theIndex的元素
virtual int indexOf(const T& theElement) const = 0;
//返回元素theElement第一次出现时的索引
virtual void erase(int theIndex) = 0;
//删除索引为theIndex的元素
virtual void insert(int theIndex, const T& theElement) = 0;
//把theElement插入线性表中索引为theIndex的位置上
virtual void output(ostream& out) const = 0;
//把线性表插入输出流out
};
arrayList.hpp
#pragma once
#include "linearList.hpp"
#include<algorithm>
#include<sstream>
#include "myExceptions.h"
template<class T>
class arrayList :public linearList<T>{
public:
//构造函数,拷贝构造函数和析构函数
arrayList(int initialCapacity = 10);
arrayList(const arrayList<T>& theList);
~arrayList() { delete[] element; }
//ADT方法
bool empty() const { return listSize == 0; }
int size() const { return listSize; }
T& get(int theIndex)const;
int indexOf(const T& theElement) const;
void erase(int theIndex);
void insert(int theIndex, const T& theElement);
void output(ostream& out) const;
//其他方法
int capacity() const { return arrayLength; }
protected:
void checkIndex(int theIndex)const;
//若索引theIndex无效,则抛出异常
T* element;//存储线性表元素的一维数组
int arrayLength;//一维数组的容量
int listSize;//线性表的元素个数
};
template<class T>
arrayList<T>::arrayList(int initialCapacity) {
if (initialCapacity < 1) {
ostringstream s;//包含头文件<sstream>
s << "Initial capacity = " << initialCapacity << "must be > 0";
throw illegalParameterValue(s.str());
}
arrayLength = initialCapacity;
element = new T[arrayLength];
listSize = 0;
}
template<class T>
arrayList<T>::arrayList(const arrayList<T>& theList) {
arrayLength = theList.arrayLength;
listSize = theList.listSize;
element = new T[arrayLength];
copy(theList.element, theList.element + listSize, element);
}
template<class T>
void arrayList<T>::checkIndex(int theIndex)const {
//确定索引theIndex在0和arrayList - 1之间
if (theIndex < 0 || theIndex > listSize - 1) {
ostringstream s;
s << "index = " << theIndex << " size = " << listSize;
throw illegalIndex(s.str());
}
}
template<class T>
T& arrayList<T>::get(int theIndex)const {
//返回索引为theIndex的元素
//若此元素不存在,则抛出异常
checkIndex(theIndex);
return element[theIndex];
}
template<class T>
int arrayList<T>::indexOf(const T& theElement) const {
//返回元素theElement第一次出现时的索引
//若该元素不存在,则返回-1
/*for (int i = 0; i < arrayList; i++) {
if (element[i] == theElement) {
return i;
break;
}
}*/
//查找元素theElement
int theIndex = (int)(find(element, element + listSize, theElement) - element);
//确定元素theElement是否找到
if(theIndex == listSize)
return -1;
return theIndex;
}
template<class T>
void arrayList<T>::erase(int theIndex) {
//删除索引为theIndex的元素
//若此元素不存在,则抛出异常illegalIndex
checkIndex(theIndex);
/*for (int i = theIndex; i < listSize - 1; i++) {
int temp = element[i];
element[i] = element[i + 1];
element[i + 1] = temp;
}
listSize--;*/
//有效索引,移动其索引大于theIndex的元素
copy(element + theIndex + 1, element + listSize, element + theIndex);
element[--listSize].~T();//调用析构函数
}
template<class T>
void changeLength1D(T* a, int oldLength, int newLength) {
if (newLength < 0) {
throw illegalParameterValue("new length must be >= 0");
}
T* temp = new T[newLength];//新数组
int number = min(oldLength, newLength);//需要复制的元素个数
copy(a, a + number, temp);
delete[] a;//释放老数组的内存空间
a = temp;
}
template<class T>
void arrayList<T>::insert(int theIndex, const T& theElement) {
//把theElement插入线性表中索引为theIndex的位置上
//if (theIndex < 0 || theIndex > listSize) {
// //无效索引
// ostringstream s;
// s << "index = " << theIndex << " size = " << listSize;
// throw illegalIndex(s.str());
//}
//有效索引,确定数组是否已满
if (listSize == arrayLength) {
changeLength1D(element, arrayLength, arrayLength * 2);
arrayLength *= 2;
}
//把元素向右移动一个位置
copy_backward(element + theIndex, element + listSize, element + listSize + 1);
element[theIndex] = theElement;
}
template<class T>
void arrayList<T>::output(ostream& out) const {
copy(element, element + listSize, ostream_iterator<T>(cout, " "));
}
template<class T>
ostream& operator<<(ostream& out, const arrayList<T>& x) {
x.output(out);
return out;
}
**main.cpp**
#include"arrayList.hpp"
int main()
{
//创建两个容量为100的线性表
linearList<int>* x = new arrayList<int>(100);
arrayList<int> y(100);
cout << y.get(2) << endl;
system("pause");
return 0;
}
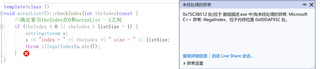
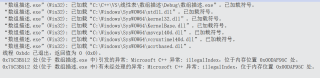