# ADD_W_FRONT i n word
def ADD_W_FRONT(i: int, n: int, word: str, textList: list) -> list:
index = i - 1
tmpStr = textList[index]
tmpList = tmpStr.strip().split()
tmpList.insert(n - 1, word)
textList[index] = ' '.join(tmpList)
return textList
# ADD_W_AFTER i n word
def ADD_W_AFTER(i: int, n: int, word: str, textList: list) -> list:
index = i - 1
tmpStr = textList[index]
tmpList = tmpStr.strip().split()
tmpList.insert(n, word)
textList[index] = ' '.join(tmpList)
return textList
# ADD_S_FRONT i sentence
def ADD_S_FRONT(i: int, sentence: str, textList: list) -> list:
index = i - 1
tmpStr = textList[index]
textList[index] = sentence + ' ' + tmpStr
return textList
# ADD_S_AFTER i sentence
def ADD_S_AFTER(i: int, sentence: str, textList: list) -> list:
index = i - 1
tmpStr = textList[index]
textList[index] = tmpStr + ' ' + sentence
return textList
# INSERT_FRONT key word
def INSERT_FRONT(key: str, word: str, textList: list) -> list:
for i in range(len(textList)):
textList[i] = textList[i].replace(key, word + ' ' + key)
return textList
# INSERT_AFTER key word
def INSERT_AFTER(key: str, word: str, textList: list) -> list:
for i in range(len(textList)):
textList[i] = textList[i].replace(key, key + ' ' + word)
return textList
# DEL_W i n
def DEL_W(i: int, n: int, textList: list) -> list:
index = i - 1
tmpStr = textList[index]
tmpList = tmpStr.strip().split()
n -= 1
if 0 <= n < len(tmpList):
del tmpList[n]
textList[index] = ' '.join(tmpList)
return textList
# DEL_L i
def DEL_L(i: int, textList: list) -> list:
index = i - 1
if 0 <= index < len(textList):
del textList[index]
return textList
# REPLACE old new
def REPLACE(old: str, new: str, textList: list) -> list:
for i in range(len(textList)):
textList[i] = textList[i].replace(old, new)
return textList
def COUNT(textList: list) -> int:
n = 0
for i in range(len(textList)):
tmpStr = textList[i]
tmpList = tmpStr.strip().split()
n += len(tmpList)
return n
def command(commandStr: str, textList: list) -> list:
# ADD_W_FRONT i n word # 在第i行中,第n個字前面加上word
# ADD_W_AFTER i n word # 在第i行中,第n個字後面加上word
# ADD_S_FRONT i sentence # 在第i行前面加入一句sentence
# ADD_S_AFTER i sentence # 在第i行後面加入一句sentence
# INSERT_FRONT key word # 在文章中所有key前面加上word
# INSERT_AFTER key word # 在文章中所有key的後面加上word
# DEL_W i n # 刪除第i行中,第n個字
# DEL_L i # 刪除第i行 (請注意其他行的行數,可能被此指令影響)
# REPLACE old new # 將文章中所有old替換為new (區分大小寫)
# COUNT # 輸出目前文章總計字數
tmpList = commandStr.strip().split()
if len(tmpList) == 0:
return textList
elif tmpList[0] == 'ADD_W_FRONT':
i, n, word = eval(tmpList[1]), eval(tmpList[2]), tmpList[3]
textList = ADD_W_FRONT(i, n, word, textList)
elif tmpList[0] == 'ADD_W_AFTER':
i, n, word = eval(tmpList[1]), eval(tmpList[2]), tmpList[3]
textList = ADD_W_AFTER(i, n, word, textList)
elif tmpList[0] == 'ADD_S_FRONT':
i, sentence = eval(tmpList[1]), ' '.join(tmpList[2:])
textList = ADD_S_FRONT(i, sentence, textList)
elif tmpList[0] == 'ADD_S_AFTER':
i, sentence = eval(tmpList[1]), ' '.join(tmpList[2:])
textList = ADD_S_AFTER(i, sentence, textList)
elif tmpList[0] == 'INSERT_FRONT':
key, word = tmpList[1], tmpList[2]
textList = INSERT_FRONT(key, word, textList)
elif tmpList[0] == 'INSERT_AFTER':
key, word = tmpList[1], tmpList[2]
textList = INSERT_AFTER(key, word, textList)
elif tmpList[0] == 'DEL_W':
i, n = eval(tmpList[1]), eval(tmpList[2])
textList = DEL_W(i, n, textList)
elif tmpList[0] == 'DEL_L':
i = eval(tmpList[1])
textList = DEL_L(i, textList)
elif tmpList[0] == 'REPLACE':
old, new = tmpList[1], tmpList[2]
textList = REPLACE(old, new, textList)
return textList
def main():
m, n = map(int, input().split())
lst = []
for _ in range(m):
lst.append(input())
num = -1
for _ in range(n):
commandStr = input().strip()
if commandStr == 'COUNT':
num = COUNT(lst)
else:
lst = command(commandStr, lst)
if num != -1:
print(num)
for line in lst:
print(line)
if __name__ == '__main__':
main()
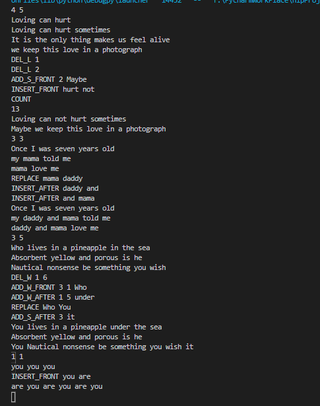