package com.example.dan1244;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteDatabase.CursorFactory;
import android.database.sqlite.SQLiteOpenHelper;
import android.widget.Toast;
public class MyDB {
public SQLiteDatabase db=null; //数据库类
private final static String DATABASE_NAME= "db1.db";//数据库名称
private final static String TABLE_NAME="table01"; //数据表名称
private final static String _ID = "_id"; //数据表字段/
private final static String NAME = "name";
private final static String PRICE = "price";
private final static String NO = "no";
/*建立数据表的字段*/
private final static String CREATE_TABLE = "CREATE TABLE " + TABLE_NAME + " (" + _ID
+ " integer primary key autoincrement," + NAME + " text,"+ NO + " text);";
private Context mCtx = null;
/**
public MyDB(Context ctx,String name,CursorFactory factory,int version)
{//构造函数
super(ctx,name,factory,version);
this.mCtx=ctx; //传入建立对象的Activity
}
public void onCreate(SQLiteDatabase db)
{
db.execSQL(CREATE_TABLE);
Toast.makeText(mCtx,"创建成功!",Toast.LENGTH_LONG).show();
}
public void onUpgrade(SQLiteDatabase db,int oldVersion,int newVersion)
{
}**/
public void open() throws SQLException {
//打开已经存在的数据库
//super.onOpen(db);
db = mCtx.openOrCreateDatabase(DATABASE_NAME, 0, null);
try {
db.execSQL(CREATE_TABLE);//建立数据表
}catch (Exception e) {
Toast.makeText(mCtx,"创建失败!",Toast.LENGTH_LONG).show();
}
}
public void close() { //关闭数据库
db.close();
}
// public Cursor getAll() { //查询所有数据,取出所有的字段
// return db.rawQuery("SELECT * FROM " + TABLE_NAME, null);
// }
public Cursor getAll() { //查询所有数据,只取出三个字段
return db.query(TABLE_NAME,
new String[] {_ID, NAME, PRICE},
null, null, null, null, null,null);
}
// public Cursor get(long rowId) throws SQLException { // 查询指定ID的数据,只取出三个字段
// Cursor mCursor = db.query(TABLE_NAME,
// new String[] {_ID, NAME, PRICE},
// _ID +"=" + rowId, null, null, null, null,null);
// if (mCursor != null) {
// mCursor.moveToFirst();
// }
// return mCursor;
// }
public long append(String no,String name) { //新增一条记录
ContentValues args = new ContentValues();
args.put(NO, no);
args.put(NAME, name);
return db.insert(TABLE_NAME, null, args);
}
public boolean delete(long rowId) { //删除指定的数据
return db.delete(TABLE_NAME, _ID + "=" + rowId, null) > 0;
}
public boolean update(long rowId, String name,int price) { //更新指定的数据
ContentValues args = new ContentValues();
args.put(NAME, name);
args.put(PRICE, price);
return db.update(TABLE_NAME, args,_ID + "=" + rowId, null) > 0;
}
public void droptable() {
// TODO Auto-generated method stub
db.execSQL("DROP TABLE "+TABLE_NAME);
}
public Cursor get(String s) throws SQLException {
// TODO Auto-generated method stub
Cursor mCursor = db.query(TABLE_NAME,
new String[] {_ID, NAME, NO},
NO +"=" + "'"+s+"'", null, null, null, null,null);
return mCursor;
}
}
package com.example.dan1244;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import android.app.Activity;
import android.content.Intent;
import android.database.Cursor;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Toast;
public class MainActivity extends Activity implements OnClickListener {
private MyDB db=null;
Cursor cursor;
Button bu1;
Button bu2;
EditText ed1, ed2, ed3;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bu1=(Button) findViewById(R.id.button1);
bu2=(Button) findViewById(R.id.button2);
ed1=(EditText) findViewById(R.id.editText1);
ed2=(EditText) findViewById(R.id.editText2);
ed3=(EditText) findViewById(R.id.editText3);
bu1.setOnClickListener(this);
bu2.setOnClickListener(this);
db=new MyDB();
db.open();
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
try {
switch (v.getId()) {
case R.id.button1:
String dc=ed1.getText().toString();
String js=ed2.getText().toString();
db.append(dc,js);
Toast.makeText(MainActivity.this, "添加成功", Toast.LENGTH_LONG).show();
break;
case R.id.button2:
String s=ed3.getText().toString();
cursor=db.get(s);
Bundle data=new Bundle();
data.putSerializable("data", converCursorToList(cursor));
Intent i=new Intent(MainActivity.this, Sdier.class);
i.putExtras(data);
startActivity(i);
break;
}
} catch (Exception e) {
// TODO: handle exception
Toast.makeText(MainActivity.this, "失败", Toast.LENGTH_LONG).show();
Log.e("--------", e.getMessage());
}
}
protected ArrayList<Map<String, String> > converCursorToList(Cursor cursor){
ArrayList<Map<String, String>> result = new ArrayList<Map<String,String>>();
//遍历Cursor结果集
while(cursor.moveToNext()){
//将结果集集中地数据存入Arraylist中
Map<String,String> map = new HashMap<String, String>();
map.put("name", cursor.getString(1));
map.put("no", cursor.getString(2));
result.add(map);
}
return result;
}
@Override
protected void onDestroy() {
super.onDestroy();
db.droptable();
db.close();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
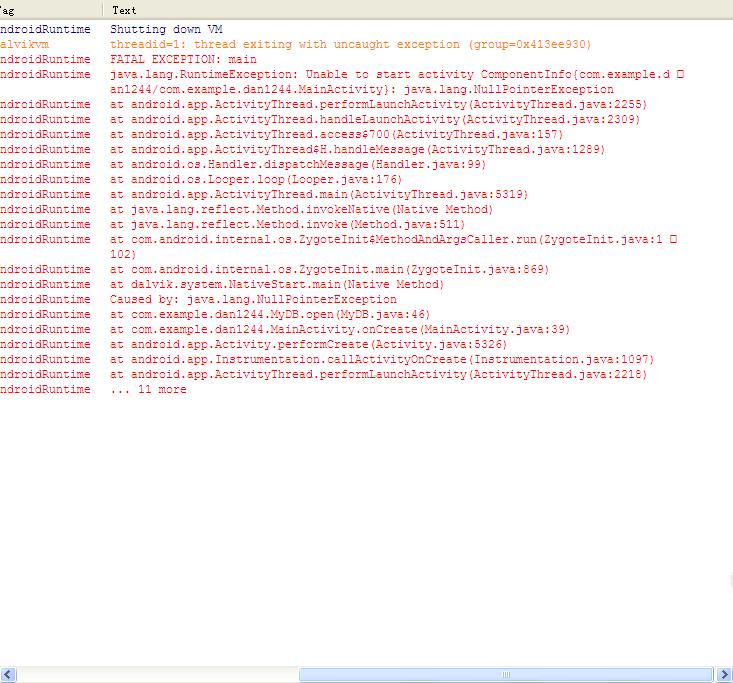