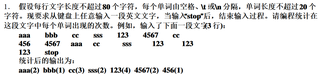
#include <stdio.h>
#include<stdlib.h>
#include <string.h>
struct node
{
int count;
char data [20];
struct node *next;
};
struct node *create ()
{
char str[20] ;
struct node *head,*r, *s, *p;
head=NULL;
printf ("输入字符串(以stop标记结束) :\n") ;
while(1)
{
scanf ("%s",str) ;
if(strcmp(str, "stop")==0)
break;
p=head;
while(p!=NULL&&strcmp(p->data,str)!=0)
p=p->next;
if(p!=NULL)
p->count++;
else
{
s=(struct node* )malloc(sizeof(node));
s->count=1;
strcpy(s->data,str);
s->next=NULL;
if(head=NULL)
{
head=s;
r=s;
}
else
{
r->next=s;
r=s;
}
}
}
return head;
}
void disp(struct node *p)
{
if(p==NULL)
printf("空表\n");
else
while(p!=NULL)
{
printf("%s(%d) ",p->data,p->count);
p=p->next;
}
printf("\n");
}
int main()
{
struct node *p;
p=create();
printf("输出单链表:\n");
disp(p);
return 0;
}