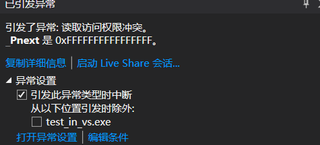
#include <iostream>
#include <string>
using namespace std;
struct student {
string name;
int score{};
};
struct teacher {
string name;
student stu_arr[5];
};
void assign(teacher* p, string tch_name[3], string stu_name[3][5], int scores[3][5])
{
for (int i = 0; i < 3; i++)
{
p->name = tch_name[i];
for (int j = 0; j < 5; j++)
{
p->stu_arr[j].name = stu_name[i][j];
p->stu_arr[j].score = scores[i][j];
}
}
return;
}
int main()
{
teacher tch_arr[3];
string tch_name[3] = { "老李","老王","老张" };
string stu_name[3][5] =
{
{ "1张三","1李四","1王五","1赵六","1周七" },
{ "2张三","2李四","2王五","2赵六","2周七" },
{ "3张三","3李四","3王五","3赵六","3周七" }
};
int scores[3][5] =
{
{ 50, 60, 70, 80, 90 },
{ 78, 97, 55, 65, 85 },
{ 85, 62, 43, 66, 90 }
};
assign(tch_arr, tch_name, stu_name, scores);
for (int i = 0; i < 3; i++)
{
cout << tch_arr[i].name << "老师的学生成绩如下:" << endl;
for (int j = 0; j < 5; j++)
{
cout << tch_arr[i].stu_arr[j].name << ": " << tch_arr[i].stu_arr[j].score << "\t";
}
cout << endl;
}
system("pause");
return 0;
}
_CONSTEXPR20_CONTAINER void _Container_base12::_Orphan_all_unlocked() noexcept {
for (auto& _Pnext = _Myproxy->_Myfirstiter; _Pnext; _Pnext = _Pnext->_Mynextiter) {
_Pnext->_Myproxy = nullptr;
}
_Myproxy->_Myfirstiter = nullptr;
}