private static int BUFFER = 1024 * 4; // 缓冲大小
private static byte[] B_ARRAY = new byte[BUFFER];
/**
* 压缩文件
* @param sourceDir
* @param zipFile
*/
public static void zip(String sourceDir, String zipFile) throws Exception
{
OutputStream os = null;
BufferedOutputStream bos = null;
ZipOutputStream zos = null;
try
{
File zip = new File(zipFile);
if (!zip.getParentFile().exists())
zip.getParentFile().mkdirs();
os = new FileOutputStream(zipFile);
bos = new BufferedOutputStream(os);
zos = new ZipOutputStream(bos);
File file = new File(sourceDir);
String basePath = null;
if (file.isDirectory())
{
basePath = file.getPath();
}
else
{
basePath = file.getParent();
}
basePath = basePath+""+File.separatorChar;
zipFile(file, basePath, zos);
zos.closeEntry();
}
catch (Exception e)
{
new BaseBean().writeLog("压缩文件出错:"+e);
throw e;
}
finally
{
try
{
if(null!=zos)
{
zos.close();
}
}
catch(Exception e)
{
}
try
{
if(null!=bos)
{
bos.close();
}
}
catch(Exception e)
{
}
try
{
if(null!=os)
{
os.close();
}
}
catch(Exception e)
{
}
}
}
/**
* 递归压缩目录下的所有文件
* @param source
* @param basePath
* @param zos
*/
private static void zipFile(File source, String basePath, ZipOutputStream zos) throws Exception
{
File[] files = new File[0];
if (source.isDirectory())
{
files = source.listFiles();
}
else
{
files = new File[1];
files[0] = source;
}
String pathName;
byte[] buf = new byte[1024];
int length = 0;
try
{
for (File file : files)
{
if (file.isDirectory())
{
pathName = file.getPath().substring(basePath.length())+""+File.separatorChar;
//System.out.println("pathName 1 : "+pathName);
//zos.putNextEntry(new ZipEntry(pathName));
zipFile(file, basePath, zos);
}
else
{
pathName = file.getPath().substring(basePath.length());
ZipEntry zipEntry = new ZipEntry(pathName);
zipEntry.setSize(file.length());
zipEntry.setTime(file.lastModified());
//System.out.println("pathName 2 : "+pathName);
InputStream is = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(is);
zos.putNextEntry(zipEntry);
while ((length = bis.read(buf)) > 0)
{
zos.write(buf, 0, length);
}
bis.close();
is.close();
}
}
}
catch (Exception e)
{
e.printStackTrace();
new BaseBean().writeLog("压缩文件出错 zipFile : "+e);
throw e;
}
}
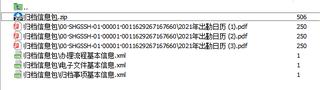