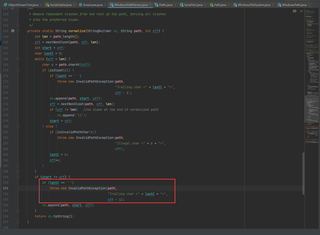
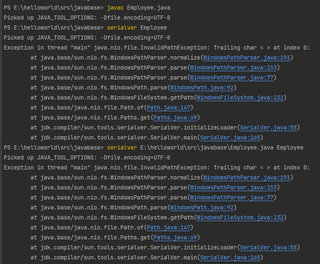
package javabase;
import java.io.Serializable;
import java.time.LocalDate;
import java.util.*;
public class Employee implements Serializable {
private String name;
private double salary;
private LocalDate hireDay;
public static final int NAME_SIZE=80;
public static final int RECORD_SIZE=100;
public String getName()
{
return name;
}
public String getDescription()
{
return String.format("an employee with a salary of $%.2f",salary);
}
public Employee(String name,double salary,int year,int month,int day)
{
this.name=name;
this.salary=salary;
hireDay=LocalDate.of(year,month,day);
}
public Employee(String name)
{
this.name=name;
}
public Employee()
{}
public double getSalary() {
return salary;
}
public LocalDate getHireDay()
{
return hireDay;
}
public void raiseSalary(double byPercent)
{
double raise=this.salary*byPercent/100;
this.salary+=raise;
}
@Override
public boolean equals(Object otherObject)
{
if(this==otherObject) {
return true;
}
System.out.println("\n\n身份不同");
if (otherObject==null) {
return false;
}
if (getClass()!=otherObject.getClass()) {
return false;
}
var other=(Employee)otherObject;
return Objects.equals(name,other.name)
&&salary==other.salary
&&Objects.equals(hireDay,other.hireDay);
}
public int hashcode()
{
return Objects.hash(name,salary,hireDay);
}
@Override
public String toString() {
return "Employee{" +
"name='" + name + '\'' +
", salary=" + salary +
", hireDay=" + hireDay +
'}';
}
}