#include "stdio.h"
#include "stdlib.h"
#include "assert.h"
typedef struct student {
char Name[20];
int StuID;
int Grade;
}stu;
//创建表
struct Node {
struct student data;
struct Node* next;
};
//创建表头
struct Node* createList() {
struct Node* headNode = (struct Node*)malloc(sizeof(struct Node));
assert(headNode);
headNode->next = NULL;
return headNode;
}
//创建结点
struct Node* createNode(struct student data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
assert(newNode);
newNode->data = data;
newNode->next = NULL;
return newNode;
}
//指定节点删除
void deleteAppointNodeByID(struct Node* headNode,int posID) {
struct Node* posNode = headNode->next;
struct Node* posFrontNode = headNode;
if (posNode == NULL) {
printf("数据为空,无法删除!\n");
return;
}
while (posNode->data.StuID = posID) {
posFrontNode = posNode;
posNode = posFrontNode->next;
if (posNode == NULL) {
printf("未找到指定位置,无法删除!\n");
return;
}
}
posFrontNode->next = posNode->next;
free(posNode);
}
//查找功能
struct Node* searchInfoByData(struct Node* headNode, int posData) {
struct Node* posNode = headNode;
while (posNode!=NULL) {
if (posNode->data.StuID == posData)
return posNode;
posNode = posNode->next;
}
printf("无法找到结点\n");
return NULL;
}
//插入结点
void insertNodeByHead(struct Node* headNode, struct student data) {
struct Node* newNode = createNode(data);
newNode->next = headNode->next;
headNode->next = newNode;
}
//打印链表
void printList(struct Node* headNode) {
struct Node* pMove = headNode->next;
printf("Name\tStuID\tGrade");
while (pMove) {
printf("%s\t%d\t%d",pMove->data.Name,pMove->data.StuID,pMove->data.Grade);
pMove = pMove->next;
}
printf("\n");
}
//建表
//按键交互
void keyDown() {
struct Node* list = createList();
struct student data;
printf("请输入:(0`4)\n");
switch (getchar()) {
case '0':
printf("正常退出!\n");
system("pause");
exit(0);
break;
case '1':
printf("录入信息:\n");
//插入链表
printf("请输入学生姓名,学号,成绩\n");
fflush(stdin);
scanf_s("%s%d%d",data.Name,4,&data.StuID,&data.Grade);
insertNodeByHead(list,data);
break;
case '2':
printf("浏览信息:\n");
printList(list);
break;
case '3':
printf("删除信息:\n");
break;
case '4':
printf("查找信息:\n");
break;
default:
printf("输入错误,请重新输入!\n");
break;
}
}
//菜单
void menu() {
printf("----------------------------\n");
printf("学生信息管理系统\n");
printf("1.录入信息\n");
printf("2.浏览信息\n");
printf("3.删除信息\n");
printf("4.查找信息\n");
printf("0.退出系统\n");
printf("----------------------------\n");
}
int main() {
while (1) {
menu();
keyDown();
system("pause");
system("cls");
}
system("pause");
return 0;
}
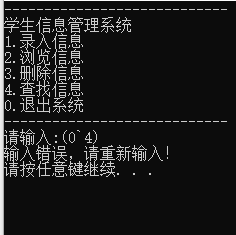