package com.service;
public interface StudentService {
void put(String key, String value);
}
package com.service.impl;
import com.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private RedisTemplate<Object,Object> redisTemplate;
@Override
public void put(String key, String value) {
redisTemplate.opsForValue().set(key,value);
}
}
package com.web;
import com.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
@RequestMapping(value = "/put")
@ResponseBody
public Object put(String key,String value){
studentService.put(key,value);
return "值已成功放入redis";
}
}
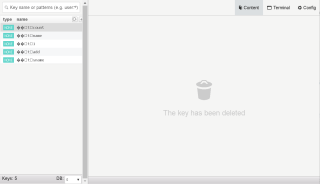