问题遇到的现象和发生背景
用代码块功能插入代码,请勿粘贴截图
我想要达到的结果
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.home {
position: absolute;
display: inline-block;
top: 30%;
left: 40%;
background-color: rgb(236, 236, 236);
padding: 20px;
border: 1px dashed black;
border-radius: 3px;
}
textarea {
box-sizing: border-box;
width: 350px;
height: 50px;
outline: none;
resize: none;
margin-bottom: 10px;
}
.item {
box-sizing: border-box;
position: relative;
border: 1px solid rgb(102, 102, 102);
border-radius: 3px;
height: 30px;
width: 350px;
background-color: rgb(255, 255, 255);
margin-bottom: 5px;
}
.changeBlue {
box-shadow: 0 0 10px rgb(109, 99, 243);
}
.item button {
position: absolute;
right: 3px;
top: 50%;
height: 20px;
margin-top: -10px;
width: 60px;
margin-right: 3px;
border: 0px;
border-radius: 3px;
background-color: red;
transition: 0.3s;
opacity: 0;
}
.item span {
line-height: 30px;
}
.item input {
line-height: 30px;
}
.footer {
margin-top: 10px;
margin-left: 0px;
}
</style>
</head>
<body>
<div class="home">
<div class="title">
<textarea class="inp"></textarea>
</div>
<div class="list">
<div class="item">
<input type="checkBox">
<span>吃饭</span>
<button class="del">删除</button>
</div>
<div class="item">
<input type="checkbox">
<span>睡觉</span>
<button class="del">删除</button>
</div>
<div class="item">
<input type="checkBox">
<span>上课</span>
<button class="del">删除</button>
</div>
</div>
<div class="footer">
<input type="checkbox" name="" id="">
<span class="total">已选0个</span>
<span>/</span>
<span class="sum">总共0个</span>
</div>
</div>
<script>
let itemList = Array.from(document.querySelectorAll('.item'))
let inp = document.querySelector('.inp')
let list = null
let total = 0;
let sum = 0;
fun()
inp.onkeydown = function (e) {
if (e.keyCode == '13') {
if (this.value.trim() == '') {
alert('输入不能为空')
event.preventDefault()
} else {
let newEle = document.createElement('div')
newEle.className = 'item'
newEle.innerHTML = `
<input type="checkBox">
<span>${this.value}</span>
<button class="del">删除</button>`
console.log(newEle);
this.parentNode.appendChild(newEle)
itemList = Array.from(document.querySelectorAll('.item'))
fun()
this.value = ''
event.preventDefault()
}
}
}
function fun() {
for (let i = 0; i < itemList.length; i++) {
itemList[i].onmouseenter = function (e) {
for (let j = 0; j < itemList.length; j++) {
if (i == j) {
} else {
itemList[j].querySelector('.del').style.opacity = '0'
}
}
let ele = document.querySelector('.changeBlue')
if (ele == null) {
} else {
ele.classList.remove('changeBlue')
}
this.classList.add('changeBlue')
this.querySelector('.del').style.opacity = '1'
}
itemList[i].onmouseleave = function () {
this.classList.remove('changeBlue')
}
}
for (let i = 0; i < itemList.length; i++) {
itemList[i].onclick = function (e) {
if (e.target.tagName == 'BUTTON') {
e.target.parentNode.parentNode.removeChild(e.target.parentNode)
}
list=document.querySelector('.list')
sum = document.querySelectorAll('.item').length
total = document.querySelectorAll('input').length-1
console.log(sum, total);
console.log(list);
}
}
sum = document.querySelectorAll('.item').length
total = document.querySelectorAll('input').length-1
console.log(sum, total);
console.log(document.querySelector('.list'));
}
</script>
</body>
</html>
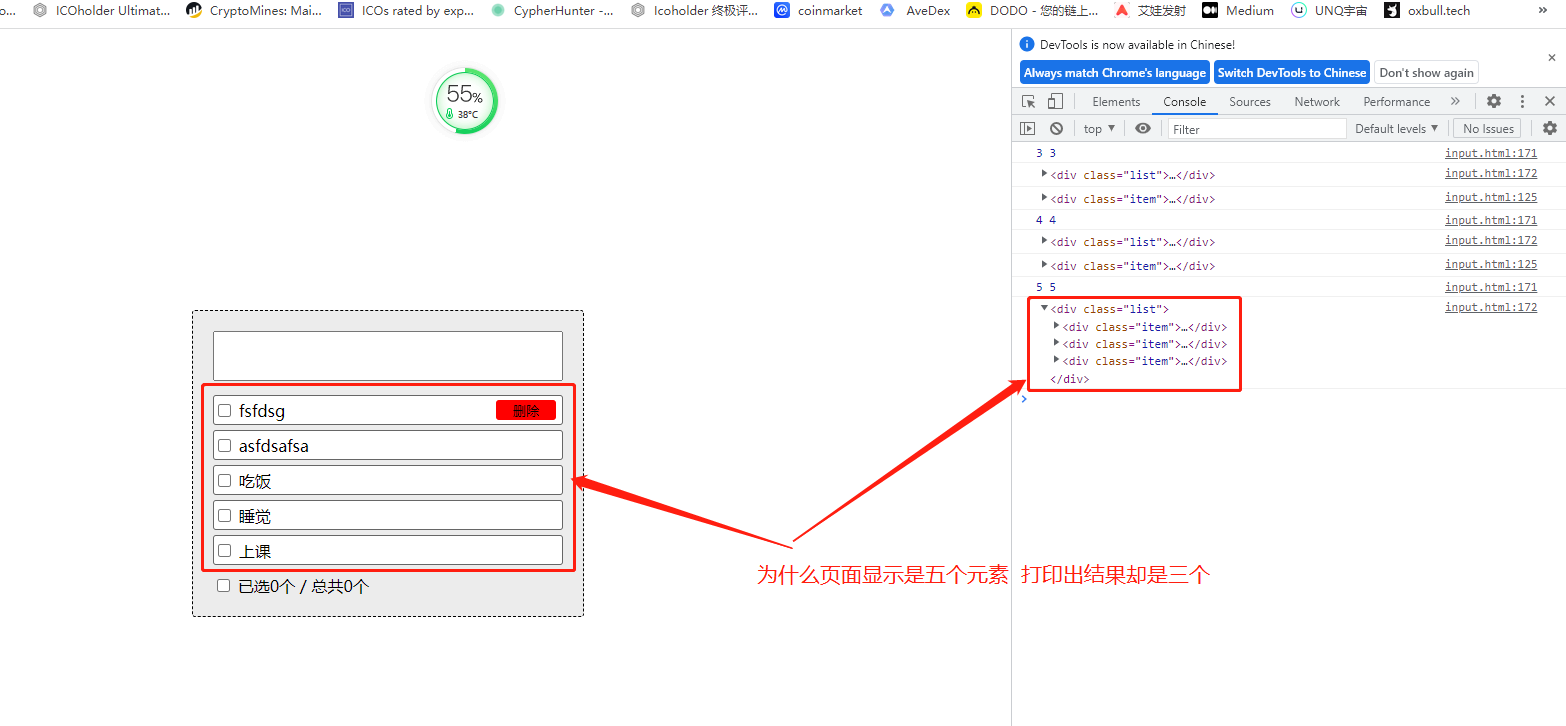
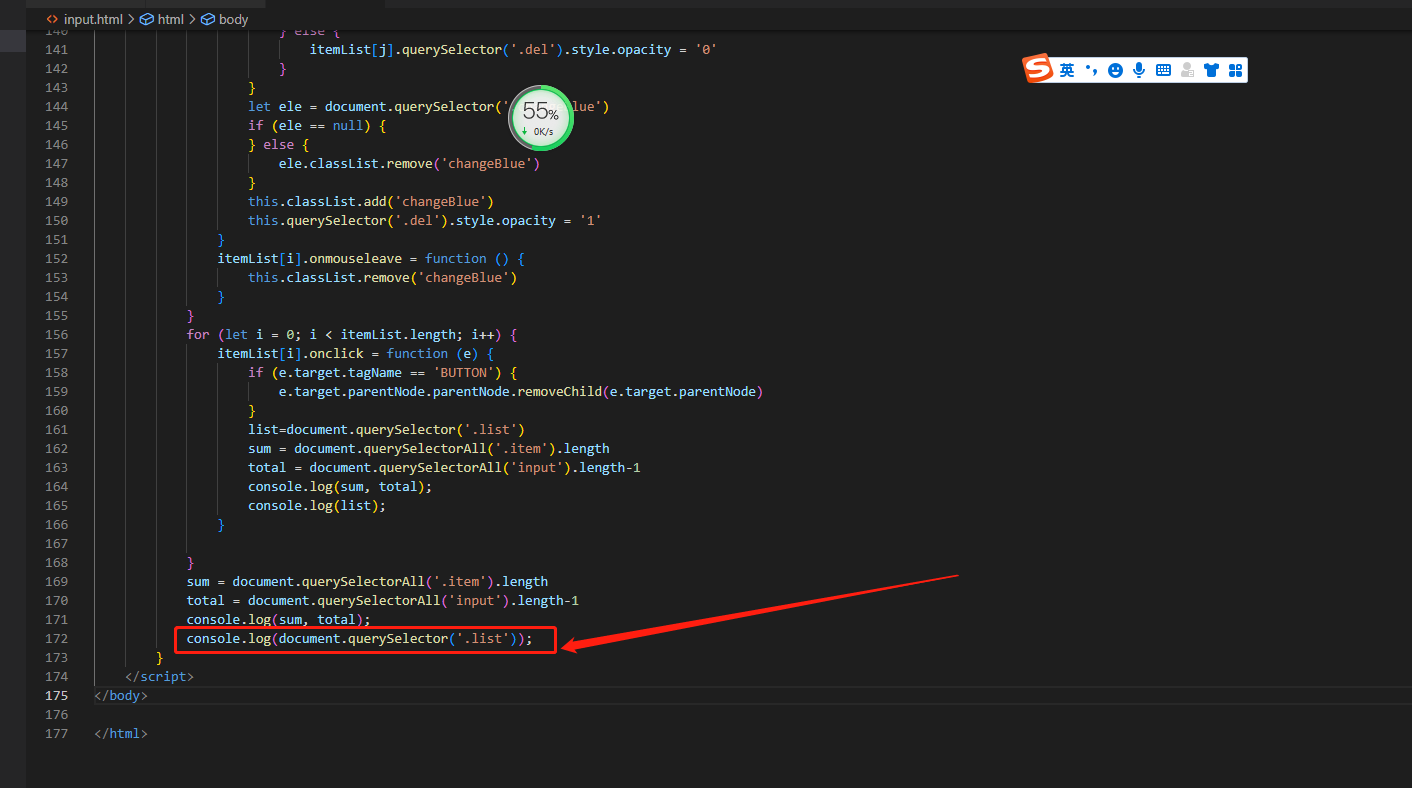


