canvas无法显示
使用一个类去绘制canvas,在浏览器元素上能显示canvas已被创建但是就是无法显示
预期效果
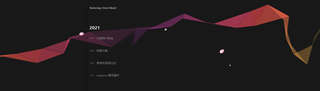
以下是原代码
<template>
<div class="RibbonAnimation" id="RibbonAnimation"></div>
</template>
<script>
// import Ribbons from './ribbon.js'
let _w = window
let _b = document.body //返回html dom中的body节点 即<body>
let _d = document.documentElement //返回html dom中的root 节点 即<html>
// random helper
let random = function () {
if (arguments.length === 1) {
// only 1 argument
if (Array.isArray(arguments[0])) {
// extract index from array
let index = Math.round(random(0, arguments[0].length - 1))
return arguments[0][index]
}
return random(0, arguments[0]) // assume numeric
} else if (arguments.length === 2) {
// two arguments range
return Math.random() * (arguments[1] - arguments[0]) + arguments[0]
}
return 0 // default
}
// screen helper
let screenInfo = function (e) {
let width = Math.max(
0,
_w.innerWidth || _d.clientWidth || _b.clientWidth || 0
),
height = Math.max(
0,
_w.innerHeight || _d.clientHeight || _b.clientHeight || 0
),
scrollx =
Math.max(0, _w.pageXOffset || _d.scrollLeft || _b.scrollLeft || 0) -
(_d.clientLeft || 0),
scrolly =
Math.max(0, _w.pageYOffset || _d.scrollTop || _b.scrollTop || 0) -
(_d.clientTop || 0)
return {
width: width,
height: height,
ratio: width / height,
centerx: width / 2,
centery: height / 2,
scrollx: scrollx,
scrolly: scrolly,
}
}
// point object
let Point = function (x, y) {
this.x = 0
this.y = 0
this.set(x, y)
}
Point.prototype = {
constructor: Point,
set: function (x, y) {
this.x = x || 0
this.y = y || 0
},
copy: function (point) {
this.x = point.x || 0
this.y = point.y || 0
return this
},
multiply: function (x, y) {
this.x *= x || 1
this.y *= y || 1
return this
},
divide: function (x, y) {
this.x /= x || 1
this.y /= y || 1
return this
},
add: function (x, y) {
this.x += x || 0
this.y += y || 0
return this
},
subtract: function (x, y) {
this.x -= x || 0
this.y -= y || 0
return this
},
clampX: function (min, max) {
this.x = Math.max(min, Math.min(this.x, max))
return this
},
clampY: function (min, max) {
this.y = Math.max(min, Math.min(this.y, max))
return this
},
flipX: function () {
this.x *= -1
return this
},
flipY: function () {
this.y *= -1
return this
},
}
// class prototype
class Factory {
constructor(options) {
this._canvas = null
this._context = null
this._sto = null
this._width = 0
this._height = 0
this._scroll = 0
this._ribbons = []
this._options = options
this._onDraw = this._onDraw.bind(this)
this._onResize = this._onResize.bind(this)
this._onScroll = this._onScroll.bind(this)
this.setOptions(options)
this.init()
}
// Set and merge local options
// 设置和合并本地选项
setOptions(options) {
if (typeof options === 'object') {
for (let key in options) {
if (options.hasOwnProperty(key)) {
this._options[key] = options[key]
}
}
}
}
// Initialize the ribbons effect
// 初始化丝带效果
init() {
try {
this._canvas = document.createElement('canvas')
this._canvas.style['display'] = 'block'
this._canvas.style['position'] = 'fixed'
this._canvas.style['margin'] = '0'
this._canvas.style['padding'] = '0'
this._canvas.style['border'] = '0'
this._canvas.style['outline'] = '0'
this._canvas.style['left'] = '0'
this._canvas.style['top'] = '0'
this._canvas.style['width'] = '100%'
this._canvas.style['height'] = '100%'
this._canvas.style['z-index'] = '-11'
// this._canvas.style["background-color"] = "#1f1f1f";
this._canvas.id = 'bgCanvas'
this._onResize()
this._context = this._canvas.getContext('2d')
this._context.clearRect(0, 0, this._width, this._height)
this._context.globalAlpha = this._options.colorAlpha
window.addEventListener('resize', this._onResize)
window.addEventListener('scroll', this._onScroll)
document.body.appendChild(this._canvas)
} catch (e) {
console.warn('Canvas Context Error: ' + e.toString())
return
}
this._onDraw()
}
// Create a new random ribbon and to the list
// 创建一个新的随机色带和到列表
addRibbon() {
// movement data
let dir = Math.round(random(1, 9)) > 5 ? 'right' : 'left',
stop = 1000,
hide = 200,
min = 0 - hide,
max = this._width + hide,
movex = 0,
movey = 0,
startx = dir === 'right' ? min : max,
starty = Math.round(random(0, this._height))
// asjust starty based on options
if (/^(top|min)$/i.test(this._options.verticalPosition)) {
starty = 0 + hide
} else if (/^(middle|center)$/i.test(this._options.verticalPosition)) {
starty = this._height / 2
} else if (/^(bottom|max)$/i.test(this._options.verticalPosition)) {
starty = this._height - hide
}
// ribbon sections data
let ribbon = [],
point1 = new Point(startx, starty),
point2 = new Point(startx, starty),
point3 = null,
color = Math.round(random(0, 360)),
delay = 0
// buils ribbon sections
while (true) {
if (stop <= 0) break
stop--
movex = Math.round(
(Math.random() * 1 - 0.2) * this._options.horizontalSpeed
)
movey = Math.round((Math.random() * 1 - 0.5) * (this._height * 0.25))
point3 = new Point()
point3.copy(point2)
if (dir === 'right') {
point3.add(movex, movey)
if (point2.x >= max) break
} else if (dir === 'left') {
point3.subtract(movex, movey)
if (point2.x <= min) break
}
// point3.clampY( 0, this._height );
ribbon.push({
// single ribbon section
point1: new Point(point1.x, point1.y),
point2: new Point(point2.x, point2.y),
point3: point3,
color: color,
delay: delay,
dir: dir,
alpha: 0,
phase: 0,
})
point1.copy(point2)
point2.copy(point3)
delay += 4
color += this._options.colorCycleSpeed
}
this._ribbons.push(ribbon)
}
// Draw single section
// 绘制单段
_drawRibbonSection(section) {
if (section) {
if (section.phase >= 1 && section.alpha <= 0) {
return true // done
}
if (section.delay <= 0) {
section.phase += 0.02
section.alpha = Math.sin(section.phase) * 1
section.alpha = section.alpha <= 0 ? 0 : section.alpha
section.alpha = section.alpha >= 1 ? 1 : section.alpha
if (this._options.animateSections) {
let mod = Math.sin(1 + (section.phase * Math.PI) / 2) * 0.1
if (section.dir === 'right') {
section.point1.add(mod, 0)
section.point2.add(mod, 0)
section.point3.add(mod, 0)
} else {
section.point1.subtract(mod, 0)
section.point2.subtract(mod, 0)
section.point3.subtract(mod, 0)
}
section.point1.add(0, mod)
section.point2.add(0, mod)
section.point3.add(0, mod)
}
} else {
section.delay -= 0.5
}
let s = this._options.colorSaturation,
l = this._options.colorBrightness,
c =
'hsla(' +
section.color +
', ' +
s +
', ' +
l +
', ' +
section.alpha +
' )'
this._context.save()
if (this._options.parallaxAmount !== 0) {
this._context.translate(0, this._scroll * this._options.parallaxAmount)
}
this._context.beginPath()
this._context.moveTo(section.point1.x, section.point1.y)
this._context.lineTo(section.point2.x, section.point2.y)
this._context.lineTo(section.point3.x, section.point3.y)
this._context.fillStyle = c
this._context.fill()
if (this._options.strokeSize > 0) {
this._context.lineWidth = this._options.strokeSize
this._context.strokeStyle = c
this._context.lineCap = 'round'
this._context.stroke()
}
this._context.restore()
}
return false // not done yet
}
// Draw ribbons
// 绘制丝带
_onDraw() {
// cleanup on ribbons list to rtemoved finished ribbons
for (let i = 0, t = this._ribbons.length; i < t; ++i) {
if (!this._ribbons[i]) {
this._ribbons.splice(i, 1)
}
}
// draw new ribbons
this._context.clearRect(0, 0, this._width, this._height)
for (
let a = 0;
a < this._ribbons.length;
++a // single ribbon
) {
let ribbon = this._ribbons[a],
numSections = ribbon.length,
numDone = 0
for (
let b = 0;
b < numSections;
++b // ribbon section
) {
if (this._drawRibbonSection(ribbon[b])) {
numDone++ // section done
}
}
if (numDone >= numSections) {
// ribbon done
this._ribbons[a] = null
}
}
// maintain optional number of ribbons on canvas
if (this._ribbons.length < this._options.ribbonCount) {
this.addRibbon()
}
requestAnimationFrame(this._onDraw)
}
// Update container size info
// 更新容器大小信息
_onResize(e) {
let screen = screenInfo(e)
this._width = screen.width
this._height = screen.height
if (this._canvas) {
this._canvas.width = this._width
this._canvas.height = this._height
if (this._context) {
this._context.globalAlpha = this._options.colorAlpha
}
}
}
// Update container size info
// 更新容器大小信息
_onScroll(e) {
let screen = screenInfo(e)
this._scroll = screen.scrolly
}
}
export default {
name: 'RibbonAnimation',
props: ['RIBBON_OPTIONS', 'RIBBONANIMATION_SHOW'],
data() {
return {
RIBBON_OPTION: this.RIBBON_OPTIONS,
visible: this.RIBBONANIMATION_SHOW,
}
},
mounted() {
if (!this.visible) {
return
}
const aaaa = new Factory(this.RIBBON_OPTION)
console.log(aaaa)
},
}
</script>
以下为Props参数
// 动态飘带参数
RibbonAnimation: {
// 动态飘带各类参数
RIBBON_OPTIONS: {
size: 90, // 默认数据
opacity: 0.6, // 透明度
zIndex: -11, // 层级
opt: {
// 色带HSL饱和度
colorSaturation: '80%',
// 色带HSL亮度量
colorBrightness: '60%',
// 带状颜色不透明度
colorAlpha: 0.65,
// 在HSL颜色空间中循环显示颜色的速度有多快
colorCycleSpeed: 6,
// 从哪一侧开始Y轴 (top|min, middle|center, bottom|max, random)
verticalPosition: 'center',
// 到达屏幕另一侧的速度有多快
horizontalSpeed: 200,
// 在任何给定时间,屏幕上会保留多少条带
ribbonCount: 5,
// 添加笔划以及色带填充颜色
strokeSize: 0,
// 通过页面滚动上的因子垂直移动色带
parallaxAmount: -0.5,
// 随着时间的推移,为每个功能区添加动画效果
animateSections: true,
},
ribbonShow: true, // 点击彩带 true显示 false为不显示
ribbonAnimationShow: true, // 滑动彩带
},
// 飘带是否显示
RIBBONANIMATION_SHOW: true,
},