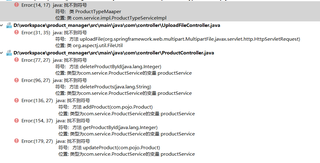
OError:(14,17)java:找不到符号
符号:类ProductTypeMaaper
位置:类com.service.impl.ProductTypeServicelmpl
D:\workspacelproduct manager\src\mainVavalcomlcontroller\UploadFileControllerjava
OError:(31,35)java:找不到符号
符号:方法uploadFile(org.springframework.web.multipart.MultipartFilejavax.servlet.http.HttpServletRequest)
位置:类org.aspectjutil.FileUtil
D:\workspacelproduct manager\srcmainVavalcomlcontroller ProductController.java
OError:(77,27)java:找不到符号
符号:方法deleteProductByldGjava.lang.lnteger)
位置:类型为com.service.ProductService的变量productService
!Error:(96,27)java:找不到符号
符号:方法deleteProductsGjava.lang.String)
位置:类型为com.service.ProductService的变量productService
Error:(136,27)java:找不到符号
符号:方法addProduct(com.pojo.Product)
位置:类型为com.service.ProductService的变量productService
Error:(154,37)java:找不到符号
符号:方法getProductByldGjava.lang.Integer)
位置:类型为com.service.ProductService的变量productService
OError:(179,27)java:找不到符号
符号:方法updateProduct(com.pojo.Product)
位置:类型为com.service.ProductService的变量productService
package com.controller;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.pojo.Product;
import com.pojo.ProductType;
import com.pojo.Supplier;
import com.service.ProductService;
import com.service.ProductTypeService;
import com.service.SupplierService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
/**
* 功能描述:商品管理的处理器
* 作者:zz
* 时间:2023/3/2 13:49
*/
@Controller
@RequestMapping("/product")
public class ProductController {
@Autowired
private ProductService productService;
@Autowired
private ProductTypeService productTypeService;
@Autowired
private SupplierService supplierService;
/**
* 方法描述:分页的查询
* 方法参数:查询条件一定是商品对象中的某一个属性,
* 返回值类型:
* 作者:zz
* 时间:2023/3/2 13:58
*/
@RequestMapping("/getProductList.action")
public String getProductList(@ModelAttribute("pro") Product product, Model model, @RequestParam(value="page",defaultValue = "1")Integer page){
System.out.println("product = " + product);
//分页查询
//pageNum:用户请求的当前页 pageSize:每页展示的记录数 3条
PageHelper.startPage(page,3);
//紧跟着第一个查询会自动分页
//查询商品的集合
List<Product> plist = productService.getProductList(product);
//创建分页对象
PageInfo<Product> pageInfo = new PageInfo<>(plist);
//把分页对象设置给model
model.addAttribute("pageInfo",pageInfo);
//查询所有的商品类型
List<ProductType> tlist = productTypeService.getTypeList();
//查询所有的供应商
List<Supplier> slist = supplierService.getSupplierList();
model.addAttribute("tlist",tlist);
model.addAttribute("slist",slist);
return "product_info/list";
}
/**
* 方法描述:根据id删除商品信息
* 方法参数:
* 返回值类型:
* 作者:zz
* 时间:2023/3/3 14:55
*/
@RequestMapping("/deleteProductById.action")
@ResponseBody
public boolean deleteProductById(Integer pid){
try {
productService.deleteProductById(pid);
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
/**
* 方法描述:批量删除
* 方法参数:6,5,4,3....
* 返回值类型:
* 作者:zz
* 时间:2023/3/3 14:55
*/
@RequestMapping("/deleteProducts.action")
@ResponseBody
public boolean deleteProducts(String pids){
try {
productService.deleteProducts(pids);
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
/**
* 方法描述:跳转商品的添加页面-查询商品类型,供应商
* 方法参数:
* 返回值类型:
* 作者:zz
* 时间:2023/3/5 8:45
*/
@RequestMapping("/toAdd.action")
public String toAdd(Model model){
//查询商品的类型
List<ProductType> tlist = productTypeService.getTypeList();
//查询供应商
List<Supplier> slist = supplierService.getSupplierList();
model.addAttribute("tlist",tlist);
model.addAttribute("slist",slist);
//跳转添加页面 如果是带数据去页面,使用跳转方式转发
return "product_info/add";//正确路径:/pages/product_info/add.jsp
}
/**
* 方法描述:添加商品信息
* 方法参数:
* 返回值类型:
* 作者:zz
* 时间:2023/3/5 10:08
*/
@RequestMapping("/addProduct.action")
@ResponseBody
public boolean addProduct(Product product){
try {
System.out.println("product = " + product);
//调用业务层中添加商品方法
productService.addProduct(product);
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
/**
* 方法描述:跳转商品的修改页面-查询商品类型,供应商,根据id查询商品对象
* 方法参数:
* 返回值类型:
* 作者:zz
* 时间:2023/3/5 8:45
*/
@RequestMapping("/toUpdate.action")
public String toUpdate(Model model,Integer pid){
//根据pid查询商品对象
Product pro = productService.getProductById(pid);
//查询商品的类型
List<ProductType> tlist = productTypeService.getTypeList();
//查询供应商
List<Supplier> slist = supplierService.getSupplierList();
model.addAttribute("tlist",tlist);
model.addAttribute("slist",slist);
model.addAttribute("pro",pro);
//跳转添加页面 如果是带数据去页面,使用跳转方式转发
return "product_info/update";//正确路径:/pages/product_info/update.jsp
}
/**
* 方法描述:修改商品信息
* 方法参数:
* 返回值类型:
* 作者:zz
* 时间:2023/3/5 10:08
*/
@RequestMapping("/updateProduct.action")
@ResponseBody
public boolean updateProduct(Product product){
try {
System.out.println("product = " + product);
//调用业务层中修改商品方法
productService.updateProduct(product);
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
}