#include<malloc.h>
#include<stdio.h>
#include<stdlib.h>
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
#define OVERFLOW -2
#define STACK_INIT_SIZE 100
#define STACKINCREMENT 10
#define QueueSize 100
typedef int Status;
typedef char ElemType;
typedef struct Stack{
ElemType data[STACK_INIT_SIZE];
int top;
}SqStack;
void TraverseStack(SqStack s)
{
while(s.top!=0)
{
s.top--;
printf("s.data[%d]=%c",s.top,s.data[s.top]);
}
}
Status InitStack(SqStack&s)
{
s.top=0;
return OK;
}
Status Push(SqStack&s,ElemType e)
{
if(s.top>=0)
return ERROR;
s.data[s.top]=e;
s.top++;
return OK;
}
Status Pop(SqStack&s,ElemType &e)
{
if(s.top==0)
return ERROR;
s.top--;
e=s.data[s.top];
return OK;
}
int StackEmpty(SqStack s)
{
if(s.top==0)
return 1;
else
return 0;
}
ElemType GetTop(SqStack s)
{
if(!StackEmpty(s))
return ERROR;
ElemType e;
s.top--;
e=s.data[s.top];
return e;
}
typedef struct Queue
{
ElemType *base;
int front,rear;
}SeqQueue;
Status InitQueue(SeqQueue&Q)
{
Q.base=(ElemType*)malloc(QueueSize*sizeof(ElemType));
if(!Q.base)exit(OVERFLOW);
Q.front=Q.rear=0;
return OK;
}
Status EnQueue(SeqQueue&Q,ElemType e)
{
if((Q.rear+1)%QueueSize ==Q.front)
{
printf("Queue overflow");
return OK;
}
Q.base[Q.rear]=e;
Q.rear=(Q.rear+1)%QueueSize;
return OK;
}
Status DeQueue(SeqQueue&Q,ElemType &e)
{
if(Q.front==Q.rear)
{
printf("Queue empty");
return ERROR;
}
e=Q.base[Q.front];
Q.front=(Q.front+1%QueueSize);
return OK;
}
ElemType GetHead(SeqQueue Q)
{
if(Q.front==Q.rear)
{
printf("Queue empty");
return ERROR ;
}
else return Q.base[Q.front];
}
Status QueueTraverse(SeqQueue Q)
{
int p;
if(Q.front==Q.rear){
printf("Queue empty");
return ERROR ;
}
p=Q.front;
do
{
printf("%2c",Q.base[p]);
p=(p+1)%QueueSize;
}while(p!=Q.rear);
return OK;
}
int main()
{
# SqStack s;
SeqQueue q;
ElemType ch ,e1,e2;
int state;
InitStack(s);
InitQueue(q);
printf("input a string ending by#:");
scanf("%c",&ch);
while(ch!='#')
{
Push(s,ch);
EnQueue(q,ch);
scanf("%c",&ch);
}
printf("\n The Stack is:");
TraverseStack(s);
printf("\n The Queue is:");
QueueTraverse(q);
printf("\n");
state=TRUE;
while(!StackEmpty(s)&&state)
{
if(GetTop(s)==GetHead(q))
{
Pop(s,e1);
DeQueue(q,e2);
}
else
state=FALSE;
}
if(state)
printf("This string is HuiWen!\n ");
else
printf("This string is not HuiWen!\n");
return 0;
}
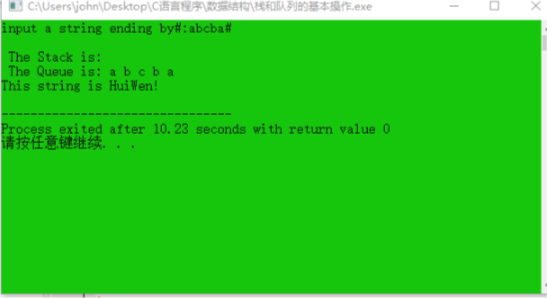
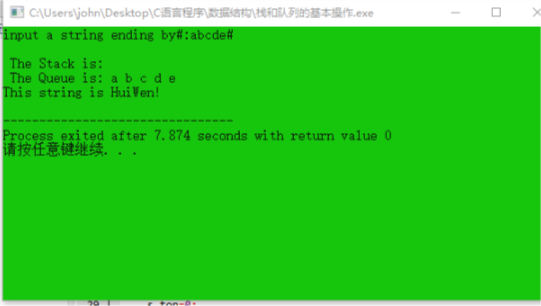