你的结果如果是--b:(3,3)的话,++和--只对实部起作用。
你的结果,最后的--b如果是--b:(3,1),++和--对实部和虚部都起作用。
两种情况的代码都贴出来了,根据你的需要选择吧。
(1)--只对实部起作用的运行结果:
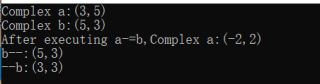
(2)--对实部和虚部都起作用的运行结果:
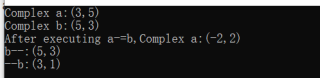
(1)的代码:(--只对实部起作用)
#include <iostream>
using namespace std;
class Complex
{
private:
int real, imag;
public:
Complex(int r = 0, int i = 0) { real = r; imag = i; }
~Complex() {}
friend ostream& operator <<(ostream& os, const Complex& c);
friend Complex& operator +=(Complex& c1, Complex& c2)
{
c1.real += c2.real;
c1.imag += c2.imag;
return c1;
}
friend Complex& operator -=(Complex& c1, Complex& c2)
{
c1.real -= c2.real;
c1.imag -= c2.imag;
return c1;
}
//前置++
Complex operator ++() {
this->real += 1;
//this->imag += 1; //如果++只对实部起作用,就注释掉这一句
return *this;
}
//后置++
Complex operator ++(int) {
Complex c(this->real, this->imag);
this->real += 1;
//this->imag += 1;//如果++只对实部起作用,就注释掉这一句
return c;
}
//前置--
Complex operator --() {
this->real -= 1;
//this->imag -= 1;//如果++只对实部起作用,就注释掉这一句
return *this;
}
//后置--
Complex operator --(int) {
Complex c(this->real, this->imag);
this->real -= 1;
//this->imag -= 1;//如果++只对实部起作用,就注释掉这一句
return c;
}
};
ostream& operator <<(ostream& os, const Complex& c)
{
os << "(" << c.real << "," << c.imag << ")";
return os;
}
int main()
{
Complex a(3, 5), b(5, 3);
cout << "Complex a:" << a << endl;
cout << "Complex b:" << b << endl;
a -= b;
cout << "After executing a-=b,Complex a:" << a << endl;
cout << "b--:" << b-- << endl;
cout << "--b:" << --b << endl;
return 0;
}
(2)的代码:(--对实部和虚部都起作用)
#include <iostream>
using namespace std;
class Complex
{
private:
int real, imag;
public:
Complex(int r=0,int i = 0) { real = r; imag = i; }
~Complex() {}
friend ostream& operator <<(ostream& os, const Complex& c);
friend Complex& operator +=(Complex& c1, Complex& c2)
{
c1.real += c2.real;
c1.imag += c2.imag;
return c1;
}
friend Complex& operator -=(Complex& c1, Complex& c2)
{
c1.real -= c2.real;
c1.imag -= c2.imag;
return c1;
}
//前置++
Complex operator ++() {
this->real += 1;
this->imag += 1; //如果++只对实部起作用,就注释掉这一句
return *this;
}
//后置++
Complex operator ++(int) {
Complex c(this->real, this->imag);
this->real += 1;
this->imag += 1;//如果++只对实部起作用,就注释掉这一句
return c;
}
//前置--
Complex operator --() {
this->real -= 1;
this->imag -= 1;//如果++只对实部起作用,就注释掉这一句
return *this;
}
//后置--
Complex operator --(int) {
Complex c(this->real, this->imag);
this->real -= 1;
this->imag -= 1;//如果++只对实部起作用,就注释掉这一句
return c;
}
};
ostream& operator <<(ostream& os, const Complex& c)
{
os << "(" << c.real << "," << c.imag << ")";
return os;
}
int main()
{
Complex a(3, 5), b(5, 3);
cout << "Complex a:" << a << endl;
cout << "Complex b:" << b << endl;
a -= b;
cout << "After executing a-=b,Complex a:" << a << endl;
cout << "b--:" << b-- << endl;
cout << "--b:" << --b << endl;
return 0;
}