#include
using namespace std;
typedef int datatype; //结点数据类型,假设为int
typedef struct node * pointer;//结点指针类型
struct node { //结点结构
int coef; //系数
int exp; //指数
pointer next;
};
typedef pointer lklist;//单链表类型,即头指针类型
lklist initlist() { //建立一个只有头结点的空表
pointer head;
head=new node;
head->next=NULL;
return head;
}
lklist creat() {//尾插法建表,有头结点
pointer head,rear,s;
int c,e,n=0;
head=new node; //生成头结点
rear=head; //尾指针初值
cout<<"多项式的元素个数为:";
cin>>n;
for(int i=0;i<n;i++){
cout<<"输入系数coef:";
cin>>c;
cout<<"输入指数exp:";
cin>>e;
cout<<endl;
}
while(c!=0) /*输入系数为0时,表示多项式的输入结束*/
{
s=new node;
s->coef=c; /*申请新结点后赋值*/
s->exp=e; /*申请新结点后赋值*/
s->next=NULL;
rear->next=s; //尾插法
rear=s; //插入表尾,改尾指针
cout<<"系数coef:"<<c;
cout<<"指数exp:"<<e;
}
//rear->next=NULL; //尾结点的后继为空
return head;
}
/*
int n=0;
cout<<"输入初始结点数:";
cin>>n;
cout<<"输入结点数值:"<
for(int i=0;i
cin>>ch;
s=new node;
s->data=ch; //生成新结点
rear->next=s;
rear=s; //插入表尾,改尾指针
}
rear->next=NULL; //尾结点的后继为空
return head;*/
void display(lklist head){
pointer p;
p=head->next;
while(p!=head){ //OK
cout<<"为什么不出来?";
cout<coef<<"*x";
cout<<"^+"<exp;
p=p->next;
}
cout<<endl;
}
lklist add(lklist A,lklist B) { //一元多项式相加函数,用于将两个多项式相加
pointer C,p,q,rear;
int sum;
p=A->next;q=B->next;
C->next=NULL;
C=A;rear=C; //取A头结点作C头结点 //循环条件时p!=head 即p!=A
while(p!=A && q!=B)
{
if (p->exp==q->exp) //若指数相等时
{
sum=p->coef+q->coef; //相应的系数相加
if(sum!=0) //如果相加后的系数不为零 生成新结点;尾插到新链表;按x赋值新结点;A\B当前节点都前进一步
{
p->coef=sum;
C->next=p;
C=C->next;
p=p->next;
rear=q;
q=q->next;
delete rear;
}
}
else if(p->expexp) //若p指向的多项式指数小于q指向的指数
{
C->next=p; //将p结点加入到和多项式中
C=C->next;
p=p->next;
}
else //若p指向的多项式指数大于q指向的指数
{
C->next=q;
C=C->next;
q=q->next;
}
}
while(p!=A){
C=p->next;
rear=p;
p=p->next;
}
while(q!=B){
C=p->next;
rear=p;
p=p->next;
}
C=C->next;
return C;
}
int main()
{
lklist A,B;
A=initlist();
B=initlist();
cout<<"请依次输入第一个多项式的系数和指数:\n";
A=creat();
cout<<"\n";
cout<<"请依次输入第二个多项式的系数和指数:\n";
B=creat();
cout<<"输入的第一个多项式是:\n";
display(A);
cout<<"输入的第二个多项式是:\n";
display(B);
add(A,B);
cout<<"合并后的链表C:\n";
display(A);
return 0;
}
```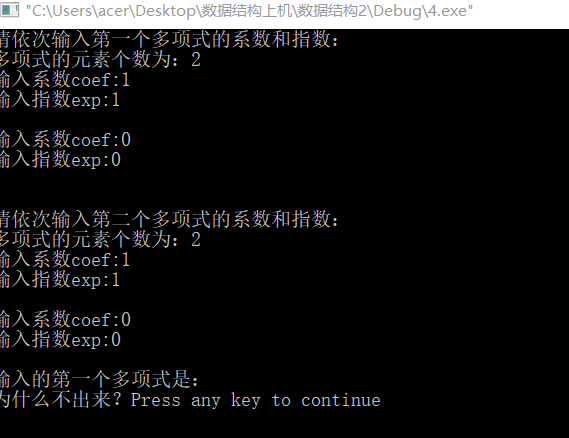
怎么办啊?为什么出不来结果。求指出哪里错误。我感觉合情合理啊