map默认是使用key排序的,因为mathScore是value中的值,需要借助vector和sort函数来实现对map的排序。
调用add函数,在map中添加数据及排序后的运行结果:
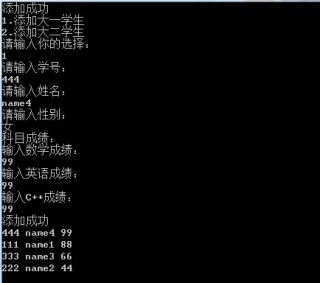
代码如下:
#include <iostream>
#include <string>
#include <map>
#include <vector>
#include <algorithm>
using namespace std;
class Stu
{
private:
string name;
int id;
string sex;
int type;
float mathscore;
float engscore;
float cppscore;
public:
Stu(){}
Stu(string n,int i,string s,float math,float eng,float cpp):name(n),id(i),sex(s),mathscore(math),engscore(eng),cppscore(cpp){}
~Stu(){}
//暂时写这几个
string getname(){return name;}
int get_id(){return id;};
float getmathscore() const {return mathscore;} //不要漏了这里的const 否则cmp函数会报错
int get_type(){return type;}
void set_type(int t){type = t;}
void change_id(int d){id = d;}
void change_name(char* nn){name = nn;}
void change_sex(char* sx){sex = sx;}
void set_mathscore(float f){mathscore = f;}
void set_engscore(float f){engscore = f;}
void set_cppscore(float f){cppscore = f;}
};
map<int,Stu>Stu_map; //声明全局变量,因为add函数中没有参数,所以把这个作为全局变量
bool cmp(const pair<int,Stu> p1,const pair<int,Stu> p2)//要用常数,不然编译错误
{
return p1.second.getmathscore() > p2.second.getmathscore();
}
istream &operator>>(istream &is,Stu &stu)
{
char temp[50];
double te;
is.getline(temp,50);
cout<<"请输入学号:"<<endl;
is.getline(temp,50);
stu.change_id(atoi(temp));
cout<<"请输入姓名:"<<endl;
is.getline(temp,20);
stu.change_name(temp);
cout<<"请输入性别:"<<endl;
is.getline(temp,10);
if(!strcmp(temp,"男")||!strcmp(temp,"女"))
{
stu.change_sex(temp);
} else
{
cout<<"性别输入错误 Σ( ° △ °|||)︴"<<endl;
}
cout<<"科目成绩:"<<endl;
cout<<"输入数学成绩:"<<endl;
cin>>te;
stu.set_mathscore(te);
cout<<"输入英语成绩:"<<endl;
cin>>te;
stu.set_engscore(te);
cout<<"输入C++成绩:"<<endl;
cin>>te;
stu.set_cppscore(te);
return is;
}
void add_stu()
{
int choice=0;
Stu stu;
cout<<"1.添加大一学生"<<endl;
cout<<"2.添加大二学生"<<endl;
cout<<"请输入你的选择:"<<endl;
cin>>choice;
switch (choice)
{
case 1:
{
cin >> stu;
stu.set_type(1);
Stu_map.insert(map<int, Stu>::value_type(stu.get_id(), stu));
cout<<"添加成功"<<endl;
break;
}
case 2:
{
cin >> stu;
stu.set_type(2);
Stu_map.insert(map<int, Stu>::value_type(stu.get_id(), stu));
cout<<"添加成功"<<endl;
break;
}
default:
break;
}
}
int main()
{
//调用函数添加4个数据
for(int i=0;i<4;i++)
add_stu();
map<int,Stu>::iterator it = Stu_map.begin();
vector< pair<int,Stu> > arr;
for (; it != Stu_map.end(); it++)
{
arr.push_back(make_pair(it->first,it->second) );
}
//排序
sort(arr.begin(),arr.end(),cmp);
//输出
vector< pair<int,Stu> >::iterator is = arr.begin();
for (; is != arr.end(); is++)
{
cout << is->first << " " << is->second.getname() << " " << is->second.getmathscore()<<endl;
}
return 0;
}