//dao
@Repository
@Mapper
public interface TeacherDao {
Teacher queryById(Integer id);
}
//mapper
<mapper namespace="com.thebugking.bugstart.dao.TeacherDao">
<resultMap id="BaseResultMap" type="com.thebugking.bugstart.bean.Teacher">
<result column="TId" jdbcType="VARCHAR" property="tid" />
<result column="Tname" jdbcType="VARCHAR" property="tname" />
</resultMap>
<select id="queryById" resultMap="BaseResultMap">
select * from Teacher where TId = #{id}
</select>
</mapper>
//service
@Service
public interface TeacherService {
Teacher queryById(Integer id);
}
@Service
public class TeacherServiceimpl implements TeacherService {
@Autowired
TeacherDao teacherDao;
@Override
public Teacher queryById(Integer id) {
Teacher teacher = teacherDao.queryById(id);
return teacher;
}
}
//control
@RestController
@RequestMapping("/")
public class TeacherController {
@Autowired
TeacherService teacherService;
@RequestMapping("get/{id}")
@ResponseBody
public Teacher GetUser(@PathVariable Integer id){
return teacherService.queryById(id);
}
}
//启动类
@SpringBootApplication
//@MapperScan("com.thrbugking.bugstart.dao")
//@ComponentScan(basePackages = {"com.thebugking.bugstart.dao"}) 加上这行不会报错,但请求不到接口报404
public class BugstartApplication {
public static void main(String[] args) {
SpringApplication.run(BugstartApplication.class, args);
}
}
//配置
server:
port: 80
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/Test?useUnicode=true & characterEncoding=utf-8 &useSSL=true & serverTimezone=Asia/Shanghai
username: root
password: root
mybatis:
mapper-locations: classpath:mapper/*.xml
type-aliases-package: com.thebugking.bugstart.bean
//报错
Error starting ApplicationContext. To display the conditions report re-run your application with 'debug' enabled.
2022-03-08 18:19:44.329 ERROR 22484 --- [ main] o.s.b.d.LoggingFailureAnalysisReporter :
***************************
APPLICATION FAILED TO START
***************************
Description:
Field teacherService in com.thebugking.bugstart.control.TeacherController required a bean of type 'com.thebugking.bugstart.service.TeacherService' that could not be found.
The injection point has the following annotations:
- @org.springframework.beans.factory.annotation.Autowired(required=true)
Action:
Consider defining a bean of type 'com.thebugking.bugstart.service.TeacherService' in your configuration.
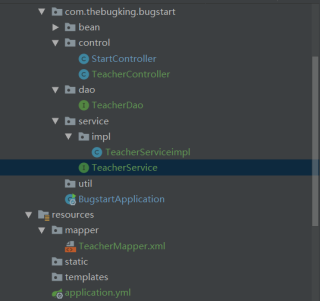