#include<stdio.h>
#include<stdlib.h>
struct std
{
char data;
struct std *lchild,*rchild;
};
int create(struct std *p)
{
char c;
scanf("%c",&c);
if(c=='#')
p=NULL;
else
{
p=(struct std*)malloc(sizeof(struct std));
p->data=c;
create(p->lchild);
create(p->rchild);
}
return 0;
};
void visit(struct std*p)
{
if(p->data!='#')
printf("%c",p->data);
}
void preorder1(struct std *p)
{
if(p!=NULL)
{
visit(p);
preorder1(p->lchild);
preorder1(p->rchild);
}
}
void midorder1(struct std *p)
{
if(p!=NULL)
{
midorder1(p->lchild);
visit(p);
midorder1(p->rchild);
}
}
int main()
{
struct std *p;
create(p);
preorder1(p);
midorder1(p);
return 0;
}
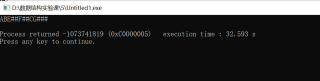