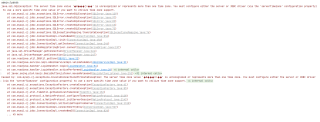
public class DBUtil {
private static final String URL = "jdbc:mysql://localhost:3306/student?useUnicode=true&characterEncoding=utf-8&useSSl=false";
private static final String DRIVER = "com.mysql.cj.jdbc.Driver";
private static final String USER_NAME = "root";
private static final String PWD = "lpb666666";
static {
try {
Class.forName(DRIVER);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConn() {
try {
return DriverManager.getConnection(URL, USER_NAME, PWD);
} catch (SQLException throwables) {
throwables.printStackTrace();
}
return null;
}
public static void closeConn(Connection connection) {
if (connection != null) {
try {
connection.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
public static void closePs(PreparedStatement ps) {
if (ps != null) {
try {
ps.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
public static void closeRs(ResultSet rs) {
if (rs != null) {
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
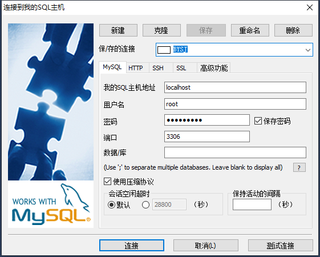
public class AdminServiceImpl implements AdminService {
public boolean validateAdmin(AdminDO adminDO) {
String userName = adminDO.getUserName();
String pwdParam = adminDO.getPwd();
String sql = "select pwd from user where user_name = ?";
Connection conn = null;
PreparedStatement ps = null;
ResultSet resultSet = null;
try {
conn = DBUtil.getConn();
if (conn == null) {
return false;
}
ps = conn.prepareStatement(sql);
ps.setString(1, userName);
resultSet = ps.executeQuery();
while (resultSet.next()) {
String pwd = resultSet.getString(1);
if (adminDO.getPwd().equals(pwd)) {
return true;
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
DBUtil.closeRs(resultSet);
DBUtil.closeConn(conn);
DBUtil.closePs(ps);
}
return false;
}
}
package com.roadjava.handler;
import com.roadjava.entity.AdminDO;
import com.roadjava.service.AdminService;
import com.roadjava.service.impl.AdminServiceImpl;
import com.roadjava.student.view.EnrolView;
import com.roadjava.student.view.LoginView;
import com.roadjava.student.view.MainView;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
public class LoginHandler extends KeyAdapter implements ActionListener {
private LoginView loginView;
public LoginHandler(LoginView loginView) {
this.loginView = loginView;
}
public void actionPerformed(ActionEvent e) {
JButton Jbutton = (JButton) e.getSource();
String text = Jbutton.getText();
if ("登录".equals(text)) {
login();
}else if ("注册".equals(text)){
new EnrolView();
}
}
private void login() {
String username = loginView.getUsernametext().getText();
char[] chars = loginView.getPasswordtext().getPassword();
if (username == null || "".equals(username.trim()) || chars == null) {
JOptionPane.showMessageDialog(loginView, "用户名密码必填");
return;
}
String password = new String(chars);
System.out.println(username + ":" + password);
if (username == null || "".equals(username.trim()) ||
password == null || "".equals(password.trim())) {
}
AdminService adminService = new AdminServiceImpl();
AdminDO adminDO = new AdminDO();
adminDO.setUserName(username);
adminDO.setPwd(password);
boolean flag = adminService.validateAdmin(adminDO);
if (flag) {
new MainView();
loginView.dispose();
} else {
JOptionPane.showMessageDialog(loginView, "用户名密码错误");
}
}
@Override
public void keyPressed(KeyEvent e) {
if (KeyEvent.VK_ENTER == e.getKeyCode()) {
login();
}
}
}