- 怎样将自定义的selector组件,放置到ProTable自带的搜索框中?
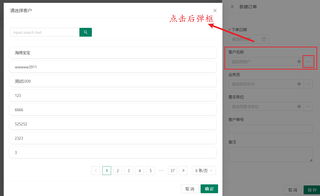
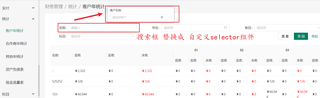
const { Search } = Input;
export default ({
data = undefined,
title,
load,
onSelected,
onBlur = undefined,
isSku = false,
fieldName = 'name',
searchName = 'name',
showPage = true,
refreshPage = false,
}) => {
const [showModal, setShowModal] = useState(false);
const pageSize = 8; //每页数量
const [page, setPage] = useState(1);
const [total, setTotal] = useState(1); //总页数
const [searchParams, setSearchParams] = useState<any>(null); //搜索
const [list, setList] = useState(null);
const [selected, setSelected] = useState<any>({});
useEffect(() => {
if (showModal) {
reload();
}
}, [page, pageSize, searchParams]);
useEffect(() => {
setSelected(data);
}, [data]);
const handleOk = () => {
console.log('handleOk', selected);
onSelected(selected || {});
//setSelected(null)
setShowModal(false);
};
const handleCancel = () => {
setSelected(null);
setShowModal(false);
};
const onClear = () => {
setSelected(null);
onSelected(null);
};
const changePage = (page) => {
setPage(page);
setList(null);
};
const onPagination = (lastPage) => {
setList(null);
changePage(lastPage);
};
const reload = () => {
const params: any = {
page,
limit: pageSize,
};
if (searchParams) {
params[searchName] = searchParams;
}
load(params).then((res) => {
unstable_batchedUpdates(() => {
if (res.list) {
setList(res.list);
setTotal(res.total);
} else {
setList(res);
}
});
});
};
const onSelect = () => {
setShowModal(true);
if (refreshPage) {
if (page != 1 || searchParams != null) {
changePage(1);
setSearchParams(null);
return;
}
}
reload();
//
};
const onSearch = (value) => {
if (searchParams != value) {
changePage(1);
setSearchParams(value);
}
};
return (
<>
<Input
className="ant-input-search"
readOnly={onBlur === undefined}
onBlur={onBlur}
placeholder={title}
addonAfter={
<Button
className="ant-input-search-button"
onClick={onSelect}
icon={<EllipsisOutlined />}
/>
}
suffix={
<>
{!!selected && (
<CloseCircleFilled
className="ant-input-clear-icon"
onClick={onClear}
/>
)}
</>
}
value={selected && selected[fieldName]}
/>
{showModal && (
<Modal
title={title}
open={true}
// onInvite={handleInvite}
onOk={handleOk}
onCancel={handleCancel}
width={800}
>
<List
className="site-card-wrapper"
grid={{ gutter: 16, column: isSku ? 4 : 1 }}
dataSource={list || []}
loading={!list}
size="large"
rowKey={'sid'}
header={
<Search
style={{ width: 300 }}
defaultValue={searchParams}
allowClear={true}
placeholder="input search text"
onSearch={(e) => {
onSearch(e.trim());
}}
enterButton
/>
}
renderItem={(item: any) => {
return (
<div
className={classNames(
styles.selectorItem,
item.sid === selected?.sid ? styles['checkCard'] : ''
)}
>
{isSku ? (
<Card
hoverable
cover={<img src={item.pic || ''} height={200} />}
onClick={() => {
setSelected(item);
}}
>
{item.sku || item.preShippingSeq}
</Card>
) : (
<Card
hoverable
className={styles.item}
onClick={() => {
setSelected(item);
}}
>
{item[fieldName]}
</Card>
)}
</div>
);
}}
pagination={
showPage && {
position: 'bottom',
size: 'default',
current: page,
// 切换页码
onChange: onPagination,
total,
pageSize,
}
}
/>
</Modal>
)}
</>
);
};
import Selector from '../../components/Selector';
const FinancialStatistics = ({ categorize, type }) => {
useEffect(() => {
loadData();
}, [page, pageSize, categorize, searchParams]);
const handleCustomer = (selected) => {
console.log('选择的客户', selected);
// setCustomerIds(selected.sid);
};
const CustomerMemo = useMemo(
() => (
<Selector
onSelected={handleCustomer}
title=‘请选择客户’
load={fetchCustomerList}
/>
),
[]
);
const loadData = () => {
const params = { categorize, year: 2023, accountId: 0, ...searchParams };
// 客户年统计
let fn;
switch (type) {
case 0:
fn = customerYearList;
break;
case 1:
fn = partnerYearList;
break;
case 2:
fn = receivableYearList;
break;
}
fn(params).then((res) => {
let totalRpValues = 0;
let totalRpedValues = 0;
let totalUnrpedValues = 0;
unstable_batchedUpdates(() => {
res.map((a) => {
a.months?.forEach((m) => {
a['rpValues' + m.month] = m.rpValues || '';
a['rpedValues' + m.month] = m.rpedValues || '';
a['unrpedValues' + m.month] =
typeof m.rpValues === 'number' && typeof m.rpedValues === 'number'
? m.rpValues - m.rpedValues
: '';
// 计算totalRpValues、totalRpedValues、totalUnrpedValues
totalRpValues += typeof m.rpValues === 'number' ? m.rpValues : 0;
totalRpedValues +=
typeof m.rpedValues === 'number' ? m.rpedValues : 0;
totalUnrpedValues +=
typeof m.rpValues === 'number' && typeof m.rpedValues === 'number'
? m.rpValues - m.rpedValues
: 0;
});
});
setData(res);
setTotal(res.length);
setTotalRpValues(totalRpValues);
setTotalRpedValues(totalRpedValues);
setTotalUnrpedValues(totalUnrpedValues);
});
});
};
const columns: any = [
{
title: '名称',
dataIndex: 'objectName',
fixed: 'left' as FixedType,
search: type != 2,
width: 100,
// renderFormItem: () => {
// return <CustomerMemo />;
// },
},
{
title: '年份',
key: 'year',
hideInTable: true,
valueType: 'dateYear',
},
// 账目列定义
{
title: '账目',
dataIndex: 'accountId',
valueEnum: accountMap,
hideInTable: true,
},
// 科目列定义
{
title: '科目',
dataIndex: 'subjectId',
valueEnum: subjectMap,
hideInTable: true,
},
{
title: string[0],
dataIndex: 'rpValues',
render: (value) => amount(value, account),
search: false,
fixed: 'left' as FixedType,
width: 150,
},
{
title: string[1],
dataIndex: 'rpedValues',
// valueType: 'money',
render: (value) => amount(value, account),
search: false,
fixed: 'left' as FixedType,
width: 150,
},
{
title: string[2],
search: false,
dataIndex: 'unrpedValues',
renderText: (_, record) => record.rpValues - record.rpedValues,
fixed: 'left' as FixedType,
render: (value) => (
<span style={{ color: 'red' }}>{amount(value, account)}</span>
),
// valueType: 'money',
width: 150,
},
];
<ProTable
search={{
defaultCollapsed: false,
}}
scroll={{ x: 3000 }}
options={false}
loading={!data}
onSubmit={onSearch}
onReset={() => onSearch({})}
rowKey="objectName"
columns={columns}
dataSource={data}