问题遇到的现象和发生背景
问题相关代码,请勿粘贴截图
运行结果及报错内容
我的解答思路和尝试过的方法
我想要达到的结果
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct student
{
char number[10];
char name[10];
int garde[3];
struct student *next;
};
struct student* head_insertion(struct student *);
struct student* tail_insertion(struct student *);
struct student* number_insertion(struct student *);
void s_printf(struct student *);
struct student* _delete (struct student *);
int main()
{
char number[10];
int choice;
char ch;
struct student *head = NULL;
struct student *prv, *corrent;
printf("Please input student information,input\\n to quit\n");
printf("intput 1 to start,q to quit:");
while ((scanf("%d",&choice))==1) //录入信息
{ printf("intput your student number:");
while ((ch = getchar()) != '\n')
{
continue;
}
gets(number);
corrent = (struct student *)malloc(sizeof(struct student));
if (head == NULL)
{
head = corrent;
}
else
prv->next = corrent;
strcpy(corrent->number, number);
printf("please input your name:");
gets(corrent->name);
printf("please input your grade:");
scanf("%d %d %d", &corrent->garde[0], &corrent->garde[1], &corrent->garde[2]);
while ((ch = getchar()) != '\n')
{
continue;
}
prv = corrent;
printf("please input next student information\n");
printf("intput 1 to start,q to quit:");
}
while ((ch = getchar()) != '\n')
{
continue;
}
printf("1.链表部插入新学生结点 2.链表尾部插入新学生结点\n");
printf("3.在某一学号学生结点之前插入新学生 4.输出该链表全部学生信息\n");
printf("5.删除指定学号的学生结点\n");
printf("请输入你要查找的功能:(输入q退出)");
while ((scanf("%d", &choice)) == 1)
{
while ((ch = getchar()) != '\n')
{
continue;
}
switch (choice)
{
case 1:
head=head_insertion(head); //链表头部插入新学生结点
break;
case 2:
head=tail_insertion(head); //链表尾部插入新学生结点
break;
case 3:
head=number_insertion(head); //在某一学号学生结点之前插入新学生
break;
case 4:
s_printf(head); //输出该链表全部学生信息
break;
case 5:
head=_delete (head); //删除指定学号的学生结点
break;
default:
break;
}
printf("请输入你要查找的功能:(输入q退出)");
}
system("pause");
return 0;
}
struct student* head_insertion(struct student *k)//表头插入
{
char ch;
struct student *put=NULL,*put2=k;
put = (struct student *)malloc(sizeof(struct student));
put->next = put2;
printf("please input your number:");
gets(put->number);
printf("please input your name:");
gets(put->name);
printf("please input your grade:");
scanf("%d %d %d", &put->garde[0], &put->garde[1], &put->garde[2]);
while ((ch = getchar()) != '\n')
{
continue;
}
put2 = put;
return k;
}
struct student* tail_insertion(struct student *k)//表尾插入
{
char ch;
struct student *put=NULL,*put2=k;
put = (struct student *)malloc(sizeof(struct student));
while (put2 != NULL)
{
put2 = put2->next;
}
put->next = put2;
printf("please input your number:");
gets(put->number);
printf("please input your name:");
gets(put->name);
printf("please input your grade:");
scanf("%d %d %d", &put->garde[0], &put->garde[1], &put->garde[2]);
while ((ch = getchar()) != '\n')
{
continue;
}
put2 = put;
return k;
}
struct student* number_insertion(struct student *k)//学号结点前插入
{
char number[10], ch;
struct student *put=NULL,*put2=k;
put = (struct student *)malloc(sizeof(struct student));
printf("please input student number:");
gets(number);
for (; put2 != NULL; put2 = put2->next)
{
if (strcmp(number, put2->number) == 0)
{
put->next = put2;
printf("please input your number:");
gets(put->number);
printf("please input your name:");
gets(put->name);
printf("please input your grade:");
scanf("%d %d %d", &put->garde[0], &put->garde[1], &put->garde[2]);
while ((ch = getchar()) != '\n')
{
continue;
}
put2 = put;
break;
}
}
return k;
}
void s_printf(struct student *k)//显示结果
{ struct student*put=k;
while (put!=NULL)
{
printf("%s %s %d %d %d\n",k->number,k->name,k->garde[0],k->garde[1],k->garde[2]);
put=put->next;
}
}
struct student* _delete (struct student *k)//删除结点
{ struct student *put=k;
char number[10];
printf("please input the number of student you want to delete:");
gets(number);
for ( ; put!=NULL; put=put->next)
{
if (strcmp(number,put->next->number)==0)
{
put->next=put->next->next;
break;
}
}
return k;
}
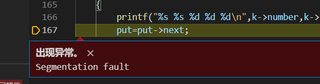