1、矩形类:
package cn.personal.demo06;
public class Rectangle {
// 定义成员变量,宽和高
private int width;
private int heigh;
// 添加有参构造方法
public Rectangle(int width, int heigh) {
super();
this.width = width;
this.heigh = heigh;
}
// 添加get/set方法
public int getWidth() {
return width;
}
public void setWidth(int width) {
this.width = width;
}
public int getHeigh() {
return heigh;
}
public void setHeigh(int heigh) {
this.heigh = heigh;
}
//获取面积的方法
public int getArea(int wi,int he) {
return wi*he;
}
}
2、矩形测试类
package cn.personal.demo06;
import java.util.Arrays;
public class RectangleTest {
public static void main(String[] args) {
// 定义Rectangle数组,长度为100;
Rectangle[] rects = new Rectangle[100];
// 定义Rectangle中的width数组,长度为100;
int[] widths = new int[100];
// 定义Rectangle中的height数组,长度为100;
int[] heights = new int[100];
for (int i = 0; i < rects.length; i++) {
int wr=getRandon();
int hr=getRandon();
rects[i]=new Rectangle(wr, hr);
widths[i] = rects[i].getWidth();
heights[i] = rects[i].getHeigh();
}
//将宽度和高度进行升序排序
Arrays.sort(widths);
Arrays.sort(heights);
//循环输出打印
for (int i = 1; i <= rects.length; i++) {
System.out.println("第"+i+"大的矩形为:"
+widths[rects.length-i]+"*"+heights[rects.length-i]+"="
+rects[rects.length-i].getArea(widths[rects.length-i],heights[rects.length-i]));
}
}
public static int getRandon() {
return (int) (Math.random() * 99 + 1);
}
}
3、矩形测试效果
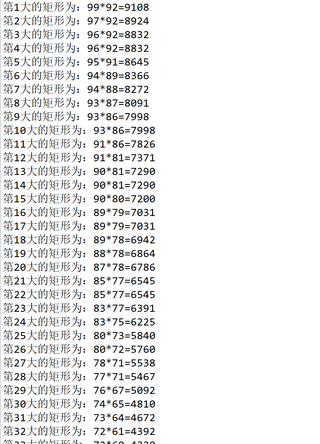
1、三角形类
package cn.personal.demo06;
public class Triangle {
//定义成员变量,三条边
private int line1;
private int line2;
private int line3;
//添加有参构造
public Triangle(int line1, int line2, int line3) {
super();
this.line1 = line1;
this.line2 = line2;
this.line3 = line3;
}
//添加get/set方法
public int getLine1() {
return line1;
}
public void setLine1(int line1) {
this.line1 = line1;
}
public int getLine2() {
return line2;
}
public void setLine2(int line2) {
this.line2 = line2;
}
public int getLine3() {
return line3;
}
public void setLine3(int line3) {
this.line3 = line3;
}
//添加求面积方法
public double getArea() {
double p = (line1 + line2 + line3) / 2d;
return Math.sqrt(p * (p - line1) * (p - line2) * (p - line3));
}
}
2、三角形测试类
package cn.personal.demo06;
public class TriangleTest {
public static void main(String[] args) {
Triangle triangle = new Triangle(3, 4, 5);
System.out.println("三边长为" + triangle.getLine1() + ","
+ triangle.getLine2() + "," + triangle.getLine3() + "的三角形面积:"
+ triangle.getArea());
triangle = new Triangle(6, 7, 8);
System.out.println("三边长为" + triangle.getLine1() + ","
+ triangle.getLine2() + "," + triangle.getLine3() + "的三角形面积:"
+ triangle.getArea());
}
}
3、三角形测试效果
