#include<stdlib.h>
#define max 100
typedef int Elemtype;
typedef struct {
Elemtype data[max];
int length;
}List;
void InitList(List *&L){
L=(List*)malloc(sizeof(List));
L->length=0;
}
int GetElem(List *L,int a,Elemtype &b){
if(a<1||a>L->length)return 0;
else b=L->data[a-1];
return 1;
}
int ListInsert(List *&L,int a,Elemtype b){
int j;
if(a<1||a>L->length)return 0;
a--;
for(j=L->length-1;j>=a;j--)L->data[j+1]=L->data[j];
L->data[a]=b;
L->length++;
return 1;
}
int ListLength(List *L){return(L->length);
}
int LocateElem(List *L,Elemtype a){
int i=0;
while(i<L->length && L->data[i]!=a)i++;
if(i>=L->length)return 0;
else return i+1;
}
void CreateList(List *&L){
int i,n;
L=(List*)malloc(sizeof(List));
printf("please input number:");
scanf("%d",&n);
printf("please input shuzu:");
for(i=0;i<n;i++)scanf("%d",&(L->data[i]));
L->length=n;
}
void DispList(List *L){
int j;
for(j=0;j<L->length;j++)printf("the outcome is:%d ",L->data[j]);
}
void unionList(List *LA,List *LB,List *&LC){
int i,len;Elemtype e;
InitList(LC);
len=ListLength(LA);
for(i=1;i<=len;i++){
GetElem(LA,i,e);
ListInsert(LC,i,e);
}
for(i=1;i<=ListLength(LB);i++){
GetElem(LB,i,e);
if(!LocateElem(LA,e))ListInsert(LC,++len,e); }
LC->length=len;
}
int main(){
List *LA,*LB,*LC;
CreateList(LA);CreateList(LB);
unionList(LA,LB,LC);
DispList(LC);
return 0;
## }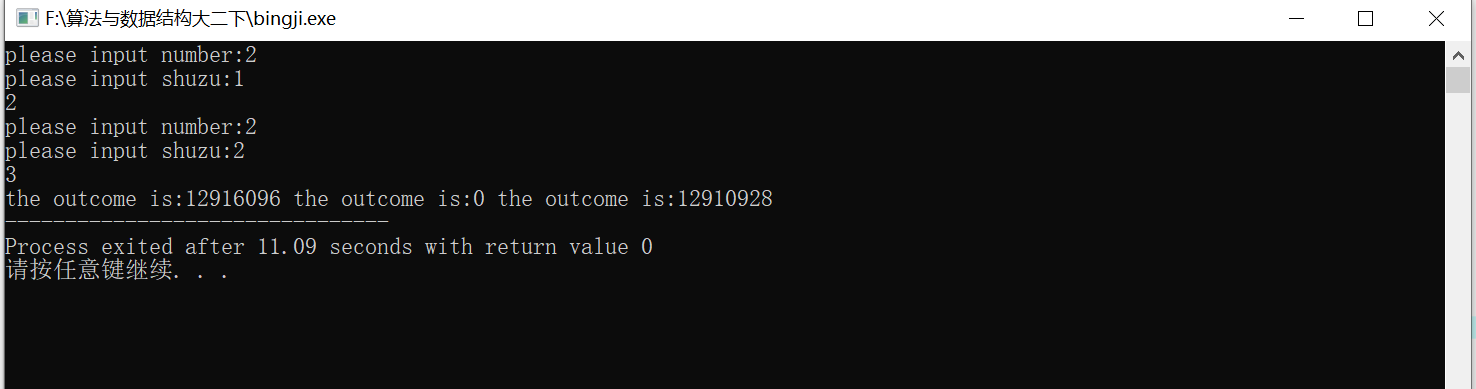