#include <stdio.h>
#include <stdlib.h>
typedef struct node
{
int data;
struct node *next;
}Node;
Node *createLink()
{
Node *head=(struct node*)malloc(sizeof(struct node));
Node *temp=head;
int i=0;
int a[10] = {1,2,3,5,7,9,10,14,15,20};
while (i<10)
{
Node *n=(struct node*)malloc(sizeof(struct node));
n->data = a[i];
n->next = NULL;
if(i==0)
{
head=temp=n;
}
else
{
temp->next = n;
temp = n;
}
i++;
}
return head;
}
Node *insert(Node *head,int data)//就是这个函数
{
Node *p=head,*pr=NULL,*pb=head;
pr=(Node *)malloc(sizeof(struct node));
if(pr==NULL)
{
printf("NO enough memory!\n");
return head;
}
pr->data=data;
pr->next=NULL;
while(p->data<data&&p->next!=NULL)
{
pb=p;
p=p->next;
}
if(p->data>=data)
{
if(p==head)
{
pr->next=head;
head=pr;
}
else
{
p=pb;
pr->next=p->next;
p->next=pr;
}
}
else
{
p->next=pr;
}
return head;
}
void Print(Node *head)
{
head=head->next;
while (head!=NULL)
{
printf("%d ", head->data);
head=head->next;
}
}
int main()
{
Node *h1=NULL;
h1=createLink();
int d;
scanf_s("%d", &d);
h1=insert(h1,d);
Print(h1);
system("pause");
return 0;
}
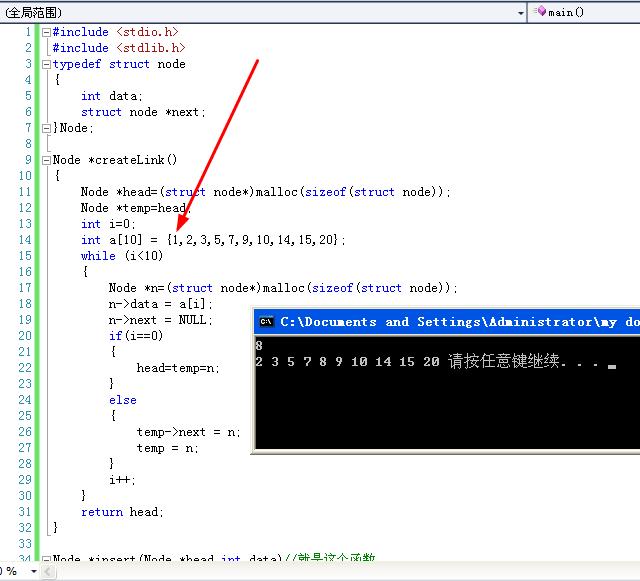