#include<iostream>
using namespace std;
struct Student
{
long num; //学号
char name[20]; //姓名
char sex; //性别
float score; //成绩
};
struct SLink
{
struct Student s;
struct SLink* next;
} *np;
void createLink()
{
np = nullptr;
}
void insertData(struct Student s)
{
if (np == nullptr){
np = (struct SLink*)malloc(sizeof(struct SLink));
np->s = s;
np->next = nullptr;
return;
}
SLink* pPrevNode = nullptr;
SLink* pNode = np;
while (pNode) {
if (s.num <= pNode->s.num) {
if (pPrevNode == nullptr) {
np = (struct SLink*)malloc(sizeof(struct SLink));
np->s = s;
np->next = pNode;
}
else {
pPrevNode->next = (struct SLink*)malloc(sizeof(struct SLink));
pPrevNode->next->s = s;
pPrevNode->next->next = pNode;
}
break;
}
if (pNode->next == nullptr){
pNode->next = (struct SLink*)malloc(sizeof(struct SLink));
pNode->next->s = s;
pNode->next->next = nullptr;
break;
}
pPrevNode = pNode;
pNode = pNode->next;
}
}
void deleteData(long num)
{
SLink* pPrevNode = nullptr;
SLink* pNode = np;
while (pNode){
if (num == pNode->s.num){
if (pPrevNode == nullptr){
np = pNode->next;
}
else{
pPrevNode->next = pNode->next;
}
//SLink* pNext = pNode->next;
free(pNode);
break;
// pNode = pNext;
}
else{
pPrevNode = pNode;
pNode = pNode->next;
}
}
}
void printStudent(const Student& stud)
{
cout << stud.num << "\t" << stud.name << "\t" << (stud.sex ? "male" : "female") << "\t" << stud.score << endl;
}
void printLink()
{
cout << "num\t" << "name\t" << "sex\t" << "score" << endl;
SLink* pNode = np;
while (pNode) {
printStudent(pNode->s);
pNode = pNode->next;
}
}
int main(void)
{
createLink();
struct Student a {0, "a", true, 100};
struct Student b {10, "b", true, 90};
struct Student c {2, "c", true, 80};
struct Student d {3, "d", true, 100};
struct Student e {43, "e", true, 99};
struct Student f {5, "f", true, 60};
insertData(a);
insertData(b);
insertData(c);
insertData(d);
insertData(e);
insertData(f);
printLink();
deleteData(10);
printLink();
int i;
cin >> i;
return 0;
}
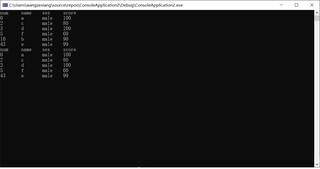