整体修改完善如下,改动见注释处,供参考:
#include <stdio.h>
#include <malloc.h>
typedef struct PolyArray
{
double coef;
int exp;
}PolyArray;
typedef struct PolyNode
{
PolyArray data;
struct PolyNode* next;
}PolyNode;
void CreatePoly(PolyNode** head, int m, PolyArray* array)
{
PolyNode* prev = NULL;
*head = (PolyNode*)malloc(sizeof(PolyNode));
(*head)->next = NULL;
for (int i = 0; i < m; i++)
{
PolyNode* p = (PolyNode*)malloc(sizeof(PolyNode));
if (p)
{
p->data.coef = array[i].coef;
p->data.exp = array[i].exp;
p->next = NULL;
if (!prev)
{
prev = *head;
}
prev->next = p;
prev = p;
}
}
}
int ComparePoly(PolyNode* a, PolyNode* b)
{
if (a->data.exp < b->data.exp)
return 1;
else if (a->data.exp > b->data.exp)
return -1;
else return 0;
}
PolyNode* AddPoly(PolyNode* ha, PolyNode* hb, PolyNode* hc)
{
PolyNode* p, * q, * newp, * newh;
p = ha->next; ha->next = NULL; //修改
q = hb->next; hb->next = NULL; //修改
newh = hc;
while (p && q)
{
switch (ComparePoly(p, q))
{
case 1:
//newp = (PolyNode*)malloc(sizeof(PolyNode)); 修改
//if (newp)
//{
//newp->data.coef = p->data.coef;
//newp->data.exp = p->data.exp;
//newp->next = NULL;
newh->next = p;//newh->next = newp;
newh = p; //newh = newp;
p = p->next;
//}
break;
case -1:
//newp = (PolyNode*)malloc(sizeof(PolyNode)); 修改
//if (newp)
//{
//newp->data.coef = q->data.coef;
//newp->data.exp = q->data.exp;
//newp->next = NULL;
newh->next = q; //newh->next = newp;
newh = q; //newh = newp;
q = q->next;
break;
//}
case 0:
double sum = p->data.coef + q->data.coef;
if (sum != 0.0)
{
//newp = (PolyNode*)malloc(sizeof(PolyNode)); 修改
//if (newp)
//{
// newp->data.coef = sum;
// newp->data.exp = q->data.exp;
// newp->next = NULL;
q->data.coef = sum;
newh->next = q;
newh = q;
q = q->next;
PolyNode* pt = p;
p = p->next;
free(pt);
// newh->next = newp;
// newh = newp;
//}
}
else { //修改
PolyNode* pt = p;
p = p->next;
free(pt);
pt = q;
q = q->next;
free(pt);
}
break;
}
}
newh->next = p ? p : q;
return hc;
}
void PrintPoly(PolyNode* head) //修改
{
FILE* fp; //修改
errno_t err; //修改
char str[] = "D:\\input.txt"; //"D:\\visualstudio\\数据结构作业2\\input.txt"
err = fopen_s(&fp, str, "at");//修改
if (err != 0) //修改
{
printf("open file error");
return;
}
PolyNode* s = head->next;
while (s)
{
fprintf(fp, "%lf %d\n", s->data.coef, s->data.exp); //修改
s = s->next;
}
fclose(fp); //修改
}
void Destory(PolyNode* head) //修改
{
PolyNode* p = NULL;
while (head) {
p = head;
head = head->next;
free(p);
}
}
int main()
{
FILE* fp;
errno_t err; //修改
char str[] = "D:\\input.txt"; //"D:\\visualstudio\\数据结构作业2\\input.txt"
err = fopen_s(&fp, str, "rt"); //修改
if (err != 0) //if (!fp) //修改
{
printf("open file error");
return 0;
}
PolyNode* ha, * hb, * hc;
PolyArray* array_a = (PolyArray*)malloc(sizeof(PolyArray) * 3);
PolyArray* array_b = (PolyArray*)malloc(sizeof(PolyArray) * 3);
if (array_a && array_b)
{
for (int i = 0; i < 3; i++)
{
fscanf_s(fp, "%lf %d\n", &array_a[i].coef, &array_a[i].exp); //修改
fscanf_s(fp, "%lf %d\n", &array_b[i].coef, &array_b[i].exp); //修改
}
}
fclose(fp); //修改
CreatePoly(&ha, 3, array_a);
CreatePoly(&hb, 3, array_b);
hc = (PolyNode*)malloc(sizeof(PolyNode));
hc->next = NULL;
AddPoly(ha, hb, hc);
PrintPoly(hc);
Destory(hc); //free(hc); 修改
Destory(ha); //free(ha); 修改
Destory(hb); //free(hb); 修改
free(array_a);
free(array_b);
return 0;
}
运行截图:
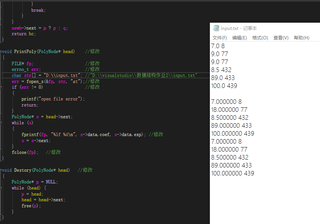