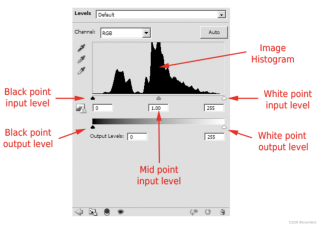
import cv2
import numpy as np
cv2.namedWindow('levels', cv2.WINDOW_NORMAL)
width = 400
height= 300
image = np.ones((height,width),dtype=np.uint8)
for num in range(width):
image[:,num ] = 0 + num / (width-1) * 255
def linear(pos,wpilv,bpilv,wpolv,bpolv):
return bpolv + (pos - bpilv) * (wpolv - bpolv) / (wpilv - bpilv)
def bpil(value):
wpilv = cv2.getTrackbarPos('White Point Input Level', 'levels')
bpilv = cv2.getTrackbarPos('Black Point Input Level', 'levels')
height,width = image.shape[0:2]
for y in range(height):
for x in range(width):
v = image[y,x]
if v <= bpilv:
image[y,x] = 0
elif v >= wpilv:
image[y,x] = 255
else:
image[y,x] = linear(v,wpilv,bpilv,255,0)
def wpil(value):
wpilv = cv2.getTrackbarPos('White Point Input Level', 'levels')
bpilv = cv2.getTrackbarPos('Black Point Input Level', 'levels')
height,width = image.shape[0:2]
for y in range(height):
for x in range(width):
v = image[y,x]
if v <= bpilv:
image[y,x] = 0
elif v >= wpilv:
image[y,x] = 255
else:
image[y,x] = linear(v,wpilv,bpilv,255,0)
cv2.createTrackbar('Black Point Input Level', 'levels', 0, 255, bpil)
cv2.createTrackbar('White Point Input Level', 'levels', 0, 255, wpil)
cv2.setTrackbarPos('White Point Input Level', 'levels', 255)
while True:
cv2.imshow('levels', image)
key = cv2.waitKey(1)
if key & 0xFF == ord('q'):
break
cv2.destroyAllWindows()
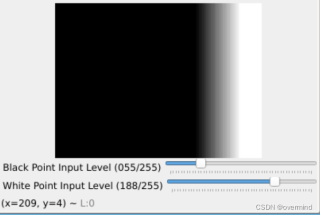
- 输入黑白场 处理成功
- 然后我准备处理输出的黑白场和中间调
import cv2
import numpy as np
cv2.namedWindow('levels', cv2.WINDOW_NORMAL)
width = 400
height= 300
image = np.ones((height,width),dtype=np.uint8)
for num in range(width):
image[:,num ] = 0 + num / (width-1) * 255
def linear(pos,wpilv,bpilv,wpolv,bpolv):
return bpolv + (pos - bpilv) * (wpolv - bpolv) / (wpilv - bpilv)
def wpil(value):
process()
def bpil(value):
process()
def wpol(value):
process()
def bpol(value):
process()
def process():
wpilv = cv2.getTrackbarPos('White Point Input Level', 'levels')
bpilv = cv2.getTrackbarPos('Black Point Input Level', 'levels')
wpolv = cv2.getTrackbarPos('White Point Output Level', 'levels')
bpolv = cv2.getTrackbarPos('Black Point Output Level', 'levels')
height,width = image.shape[0:2]
for y in range(height):
for x in range(width):
v = image[y,x]
if v <= bpilv:
image[y,x] = bpolv
elif v >= wpilv:
image[y,x] = wpolv
else:
image[y,x] = linear(v,wpilv,bpilv,wpolv,bpolv)
cv2.createTrackbar('Black Point Input Level', 'levels', 0, 255, bpil)
cv2.createTrackbar('White Point Input Level', 'levels', 0, 255, wpil)
cv2.createTrackbar('Black Point Output Level', 'levels', 0, 255,bpol)
cv2.createTrackbar('White Point Output Level', 'levels', 0, 255,wpil)
cv2.setTrackbarPos('White Point Input Level', 'levels', 255)
cv2.setTrackbarPos('White Point Output Level', 'levels', 255)
while True:
cv2.imshow('levels', image)
key = cv2.waitKey(1)
if key & 0xFF == ord('q'):
break
cv2.destroyAllWidows()
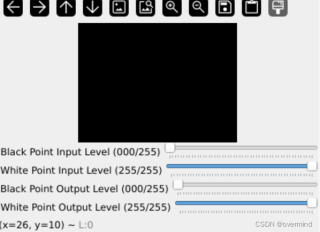
- 如何解决输入黑白场的问题
- 并且最终增加滑块 控制中间调