目录
- 使用django开发和前端界面进行交互时,提交相关数据,数据已成功提交至数据库,但是无法再前端显示。
- 我的项目/myapp/models.py
- 我的项目/myapp/views.py
- 我的项目/myapp/urls.py
- 数据库表
- 我的项目/templates/myapp/post_detail.html
- 代码
使用django开发和前端界面进行交互时,提交相关数据,数据已成功提交至数据库,但是无法再前端显示。
我的项目/myapp/models.py
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField() # 支持Markdown格式的文本
content_html = models.TextField(blank=True, editable=False) # 存储转换为HTML后的内容
author = models.ForeignKey(User, on_delete=models.CASCADE)
created_at = models.DateTimeField(auto_now_add=True)
categories = models.ManyToManyField(Category, related_name='posts')
tags = models.ManyToManyField(Tag, related_name='posts')
def __str__(self):
return self.title
我的项目/myapp/views.py
def post_detail_view(request, post_id):
# 获取帖子对象,如果不存在则返回404页面
post = get_object_or_404(Post, id=post_id)
context = {
'post': post,
'author': post.author,
'published_date': post.created_at, # 使用创建时间作为发布时间示例
'categories': post.categories.all(),
'tags': post.tags.all(),
}
return render(request, 'myapp/post_detail.html', context)
我的项目/myapp/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'), # 根路径映射到index视图函数
path('about/', views.about, name='about'), # /about/ 路径映射到about视图函数
path('contact/', views.contact, name='contact'), # /contact/ 路径映射到contact视图函数
path('login/', views.Login, name='login'), # /login/ 路径映射到user_login视图函数
path('register/', views.register, name='register'), # 注册路径映射到register视图函数
path('category/', views.category_list, name='category_list'), # /category/ 路径映射到category_list视图函数
path('post/<int:post_id>/', views.post_detail, name='post_detail'), # /post/1/ 路径映射到post_detail视图函数,带有post_id参数
path('post-list/', views.post_list, name='post_list'), # /post-list/ 路径映射到post_list视图函数
path('tag-list/', views.tag_list, name='tag_list'), # /tag-list/ 路径映射到tag_list视图函数
path('user-profile/', views.user_profile, name='user_profile'), # /user-profile/ 路径映射到user_profile视图函数
path('create/', views.create_post, name='create_post'), # /create/ 路径映射到create_post视图函数
]
数据库表
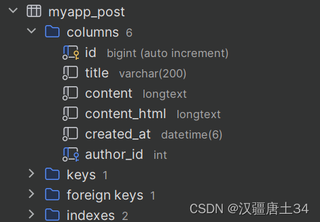

我的项目/templates/myapp/post_detail.html
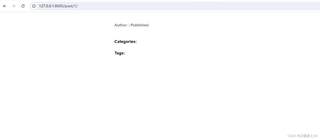
代码
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{ post.title }}</title>
<link rel="stylesheet" href="{% static 'myapp/css/styles_detail.css' %}">
<style>
body {
font-family: Arial, sans-serif;
line-height: 1.6;
margin: 0;
padding: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
}
.post-title {
font-size: 2.5em;
margin-bottom: 20px;
}
.post-meta {
font-size: 0.9em;
color: #777;
margin-bottom: 20px;
}
.post-content {
margin-bottom: 40px;
}
.post-tags, .post-categories {
list-style: none;
padding: 0;
}
.post-tags li, .post-categories li {
display: inline;
margin-right: 10px;
}
</style>
</head>
<body>
<div class="container">
<h1 class="post-title">{{ post.title }}</h1>
<p class="post-meta">
<strong>Author:</strong> {{ post.author.username }} | <!-- 使用 post.author.username 获取作者的用户名 -->
<strong>Published:</strong> {{ post.created_at|date:"Y-m-d H:i" }} <!-- 格式化显示创建时间 -->
</p>
<div class="post-content">
{{ post.content_html|safe }} <!-- 使用 safe 过滤器来渲染帖子的 HTML 内容 -->
</div>
<div class="post-categories">
<strong>Categories:</strong>
<ul>
{% for category in post.categories.all %}
<li>{{ category.name }}</li>
{% endfor %}
</ul>
</div>
<div class="post-tags">
<strong>Tags:</strong>
<ul>
{% for tag in post.tags.all %}
<li>{{ tag.name }}</li>
{% endfor %}
</ul>
</div>
</div>
</body>
</html>