一
#include<iostream>
int main()
{
float distance = 0.0f;
float height = 100;
float bounce = 0.0f;
for (int i = 0; i < 5; i++)
{
distance += height;
height /= 2;
if (i != 4)
distance += height; // 加上反弹高度
else
bounce = height; // 第五次的反弹高度
}
std::cout << "第五次落地时,共经过" << distance << "米, 反弹" << bounce << "米"<<std::endl;
}
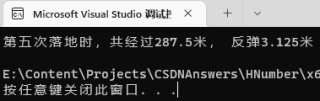
二
#include<stdlib.h>
#include<stdio.h>
struct student
{
char id[32]; // 学号
char name[64]; // 姓名
char phone[16]; // 手机
int yw_score; // 语文成绩
};
int main()
{
struct student students[5];
for (int i = 0; i < 5; ++i)
{
printf("请输入学生%d信息\n", i + 1);
printf("请输入学号:");
scanf("%s", students[i].id);
printf("请输入姓名:");
scanf("%s", students[i].name);
printf("请输入手机号:");
scanf("%s", students[i].phone);
printf("请输入语文成绩:");
scanf("%d", &students[i].yw_score);
}
for (int i = 0; i < 5; ++i)
{
printf("学生%d学号:%s,姓名:%s,手机号:%s,语文成绩:%d\n",
i + 1, students[i].id, students[i].name, students[i].phone, students[i].yw_score);
}
return 0;
}
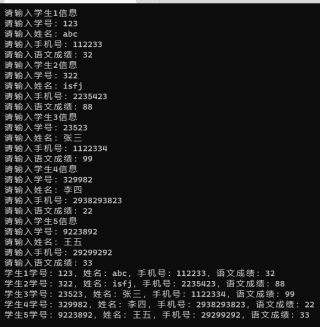
三
#include<iostream>
class box
{
public:
box() : box(1, 1, 1) {}
box(double l, double w, double h) : length(l), width(w), height(h) {}
double vol() const { return length * width * height; }
double getLength()const { return length; }
double getWidth() const { return width; }
double getHeight() const { return height; }
private:
double length;
double width;
double height;
};
int main()
{
box b1; // 调用构造函数 box()
box b2(1, 2, 3); // 调用构造函数 box(double, double, double)
// 显示对象体积
std::cout << "b1的长宽高是:" << b1.getLength() << " " << b1.getWidth() << " " << b1.getHeight() << std::endl;
std::cout << "b1的体积是:" << b1.vol() << std::endl;
std::cout << "b2的长宽高是:" << b2.getLength() << " " << b2.getWidth() << " " << b2.getHeight() << std::endl;
std::cout << "b2的体积是:" << b2.vol() << std::endl;
}
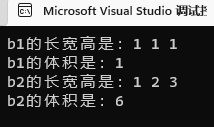
四
#include<iostream>
class score
{
public:
void setscore(double e = 100.0)
{
english = e;
}
void printscore()
{
std::cout << english;
}
private:
double english;
};
int main()
{
score stu1, stu2;
stu1.setscore(85.5);
stu2.setscore(93.5);
std::cout << "stu1的英语分数是";
stu1.printscore();
std::cout << std::endl;
std::cout << "stu2的英语分数是";
stu2.printscore();
std::cout << std::endl;
return 0;
}
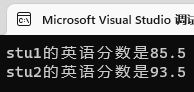