题目:1.使用设置监视哨的顺序查找在顺序表64,5,7,89,6,24里查找6,若找到,输出该元素在顺序表中的位置,否则输出提示语句“未找到”;
2.使用折半查找在顺序表4,15,17,29,56,84里查找50,若找到,输出该元素在顺序表中的位置,否则输出提示语句“未找到”。
我自己打的代码如下:
#include<stdio.h>
#include<stdlib.h>
#include<iostream>
using namespace std;
#define OK 1
#define ERROR 0
#define OVERFLOW -2
#define MAXSIZE 100
typedef int KeyType;
typedef int InfoType;
typedef struct{
KeyType key;
InfoType otherinfo;
}ElemType;
typedef struct{
ElemType *R;
int length;
}SSTable;
// 设置监视哨的顺序查找
int Search_Seq(SSTable ST,KeyType key){
int i;
ST.R[0].key=key;
for(i=ST.length;ST.R[i].key!=key;--i);
return i;
}
// 折半查找
int Search_Bin(SSTable ST,KeyType key){
int low=1;
int high=ST.length;
while(low<=high){
int mid=(low+high)/2;
if(key==ST.R[mid].key)
return mid;
else if(key<ST.R[mid].key)
high=mid-1;
else
low=mid+1;
}
return 0;
}
int main(){
int i,key;
SSTable t;
t.R=new ElemType[MAXSIZE];
cout << "请输入顺序表长度:";
cin >> t.length;
cout << "请输入您要查找的顺序表:";
for(i=1;i<=t.length;i++)
cin>>t.R[i].key;
cout<<"请输入您要查找的数据元素:";
cin>>key;
if(Search_Seq(t,key))
cout<<"该元素在顺序表中的位置为"<<Search_Seq(t,key)<<"个元素"<<endl;
else
cout<<"未找到"<<endl;
cout<<endl;
cout << "请输入顺序表长度:";
cin >> t.length;
cout << "请输入您要查找的顺序表:";
for(i=1;i<=t.length;i++)
cin>>t.R[i].key;
cout<<"请输入您要查找的数据元素:";
cin>>key;
if(Search_Bin(t,key))
cout<<"该元素在顺序表中的位置为"<<Search_Bin(t,key)<<"个元素"<<endl;
else
cout<<"未找到"<<endl;
}
代码运行结果如下图
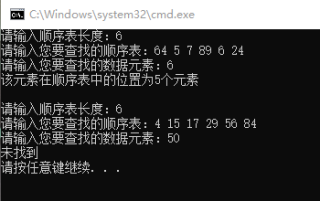
我想要达到的结果:可我觉着这个程序的提示语太多了,想简化一下主函数的代码,就是想问问怎么分别拆写顺序查找和折半查找分成两个子函数(编程要求两个查找写在同一主函数里实现调用),或许能将题中的顺序表用数组存储吗?(输出只要显示查找数据元素和查找结果的那种)怎么改写呢